New release - fpl 0.3.0
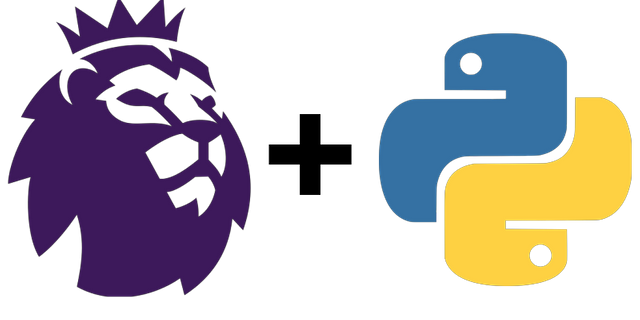
A couple of weeks ago I posted about a very basic Python wrapper I had created for the Fantasy Premier League API. In the last few days I have worked on making it a bit more user-friendly by adding classes. If you want to use the new fpl 0.3.0
you can download it from https://pypi.python.org/pypi/fpl/0.3.0 or install it with
pip install fpl
New features
Previously I had functions in the FPL
class that would return a huge JSON file, about e.g. a player, which consists of lists of dictionaries with information about said player. To get the information the person using the fpl
package would have to parse the JSON themselves, which I don't think is useful at all. So instead of making them do it all themselves I decided to create classes so that they can use that instead.
I created the following classes:
- User
- Team
- Player
- Gameweek
- ClassicLeague
- H2HLeague
I haven't done much in the past with Python classes, so it was a learning experience for me. First I thought about using the keys of the JSON returned by the Fantasy Premier League API as attributes for each class (which I implemented a rough version of two weeks ago), but then I realised that wasn't handy at all and scrapped this completely two days ago and rewrote nearly everything. This is because some of the names of the keys don't make any sense (for example a gameweek is called an event, player is called an element), so I started assigning the attributes manually to make the names more intuitive. Some keys also contain a lot of information that should be available as an attribute, so this was another reason why I chose to do it this way. Below is an example of what the Team
class looks like
class Team(object):
"""
A class representing a real team in the Fantasy Premier League.
"""
def __init__(self, team_id):
self.id = team_id
self._information = self._information()
self.name = self._information["name"]
self.short_name = self._information["short_name"]
self.current_fixture = self._information["current_event_fixture"]
self.next_fixture = self._information["next_event_fixture"]
def _information(self):
response = requests.get("{}teams".format(API_BASE_URL)).json()
return response[self.id - 1]
def __str__(self):
return self.name
So before in version 0.2.1
you would have to call the fpl.teams()
function, which returns a list of all teams (as seen here), iterate over the list to find the team you want, then finally from that you could for example get the team's name from the "name" key. This would've looked something like this
from fpl import FPL
fpl = FPL()
team = fpl.teams()[0]
print(team["name"])
>>> Arsenal
In version 0.3.0
you can simply do the following instead
from fpl.team import Team
team = Team(1)
print(team.name)
>>> Arsenal
Another example with the User
class, where in version 0.2.0
you would have to do the following to get a user's name, region and transfers for example
from fpl import FPL
fpl = FPL()
user = fpl.user(3523615)
print("{} {} - {}".format(user["entry"]["player_first_name"],
user["entry"]["player_second_name"], user["entry"]["player_region_name"])
>>> Amos Bastian - Netherlands
user_transfers = fpl.user_transfers(3523615)["history"]
print(user_transfers)
>>> ...
In version 0.3.0
you can simply do the following instead
from fpl.user import User
user = User(3523615)
print(str(user))
>>> Amos Bastian - Netherlands
print(user.transfers)
>>> ...
which is so much nicer!
How did I implement them?
The basic structure for most classes are the same, but some of them require more work than others. For example, for a Player
some information about them is available in one place (a list of all players), while more specific information requires another call to the API (for example here. To cover all this information in the Player
class I first make two calls to get all the information about a player with
def _specific(self):
"""
Returns the player with the specific player_id.
"""
return requests.get("{}element-summary/{}".format(API_BASE_URL,
self.id)).json()
def _additional(self):
"""
Returns additional information that isn't included in the other list of
players.
"""
response = requests.get("{}elements".format(API_BASE_URL)).json()
for player in response:
if player["id"] == self.id:
return player
self._specific = self._specific()
self._additional = self._additional()
then simply extract all the information to the relevant attributes of a player, like so
# Gameweek and transfer information
self.gameweek_points = self._additional["event_points"]
self.gameweek_price_change = self._additional["cost_change_event"]
self.gameweek_transfers_in = self._additional["transfers_in_event"]
self.gameweek_transfers_out = self._additional["transfers_out_event"]
self.transfers_in = self._additional["transfers_in"]
self.transfers_out = self._additional["transfers_out"]
I've also added some functions that convert magic numbers, such as a team's ID or the type of player, to the team's name and the player's position respectively, string representation for each class and changed the functions in the FPL
to return objects instead of just the JSON.
Technology
I only used Python to create the package, and the only requirement for the package itself is the Python package requests
.
Roadmap
- Add inheritance to ClassicLeague and H2HLeague classes
- Implement new classes for fixtures, player history, league standings etc.
- Implement functions so that users don't have to themselves; e.g. calculating statistics about a player like their PP90, VAPM etc.
How to contribute?
If you have any ideas about stuff you would like to see implemented you can always contact me on Discord at Amos#4622 and if you want to actually contribute you can simply submit a pull request on GitHub (or contribute in some other way, like a cool logo...)!
Posted on Utopian.io - Rewarding Open Source Contributors
Thank you for the contribution. It has been approved.
You can contact us on Discord.
[utopian-moderator]
Thanks!
Awesome piece
Hey @amosbastian I am @utopian-io. I have just upvoted you!
Achievements
Community-Driven Witness!
I am the first and only Steem Community-Driven Witness. Participate on Discord. Lets GROW TOGETHER!
Up-vote this comment to grow my power and help Open Source contributions like this one. Want to chat? Join me on Discord https://discord.gg/Pc8HG9x