SEC-S20W4 Decision Making in Programming: If-Then-Else - [ENG/UA]
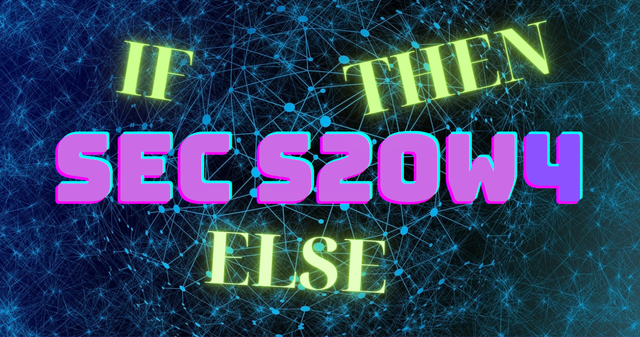
Programming is generally based on two things: conditional operators and loops. We will study the conditional operator today.
Boolean Variables. Logical Operations
Let’s start with Boolean variables. Boolean variables can store only two states - 0 and 1, but in many programming languages, they do not strictly have a numerical value. They usually represent true
or false
. At first, in languages like C/C++, there were no special Boolean variables (and values). There was zero, which was considered as false, and there was 1, which was considered true. Even more, everything that is not zero is true, and only zero is false.
In mathematics and programming, there are logical operations like >
, <
, >=
, <=
, which are obvious - but comparison operations are not so obvious. When we want to compare the values of variables a and b, we write a==b
, and it should not be confused with the assignment operation a=b
.
- The assignment operation is an action
a=b
, while - The comparison operation
a==b
is a question - perceive it this way.
Accordingly, to check for inequality, there is a!=b
.
Now about logical expressions
- these are expressions that can take the value of true or false, such as a>4
, s+3<w
, a!=78
,....
Logical expressions can be combined using logical operations - ||
(logical OR) and &&
(logical AND).
Let's say we have two expressions, and their truth values are stored in variables a
and b
. What will be the result of these expressions a||b
, a&&b
?
OR
a||b
is true when at least one of a or b is true (or both).
a||b
is false only when both are false.
This operation is called a logical OR
, or sometimes logical addition:
0 + 0 = 0
0 + 1 = 1
1 + 0 = 1
1 + 1 = 1 (since there is no "2", the truth remains true)
AND
a&&b
is true only when both values a and b are true, and false when both are false.
This operation is called logical multiplication:
0 x 0 = 0
0 x 1 = 1
1 x 0 = 1
1 x 1 = 1
NOT
The operation !
is the negation operation, which turns truth into falsehood and vice versa. So, if a=false
, then !a
will be true
and vice versa.
This is called Boolean logic or Boolean algebra. It has its laws, most of which are intuitive, but initially, it's better to avoid complex constructs.
For example, how to check if a number is divisible by 15?
If the number is divisible by 15 (exactly), the remainder will be 0.
Operator - if then else
In general, the word then
is often omitted in many programming languages, and the use is reduced to if(condition) - action for true, and action for false.
It is represented by this diagram:
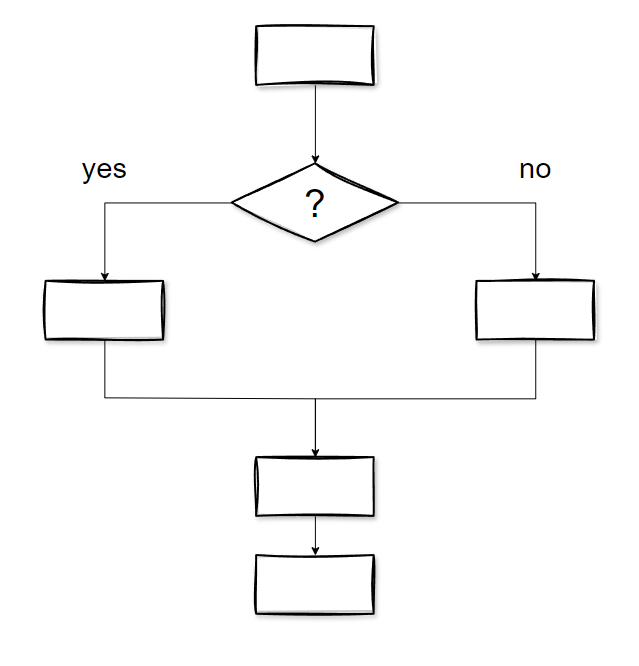
Previously, we established that commands are executed sequentially, step by step. Now, the program can either execute or skip certain actions. For example, you have $100
on your account, but someone requested to withdraw $200
. Of course, you could issue it, and it would be a credit - with the balance becoming -$100
. Or you could check this condition:
int balance=100;
int want_withdraw=200;
if(balance<withdraw)
cout<<"not enough money";
else
cout<<"Take your cash";
This code simulates behavior when withdrawing cash - if the balance is less than the requested amount, the ATM checks this condition and makes a decision based on it.
A conditional operator can be incomplete - without the else
part:
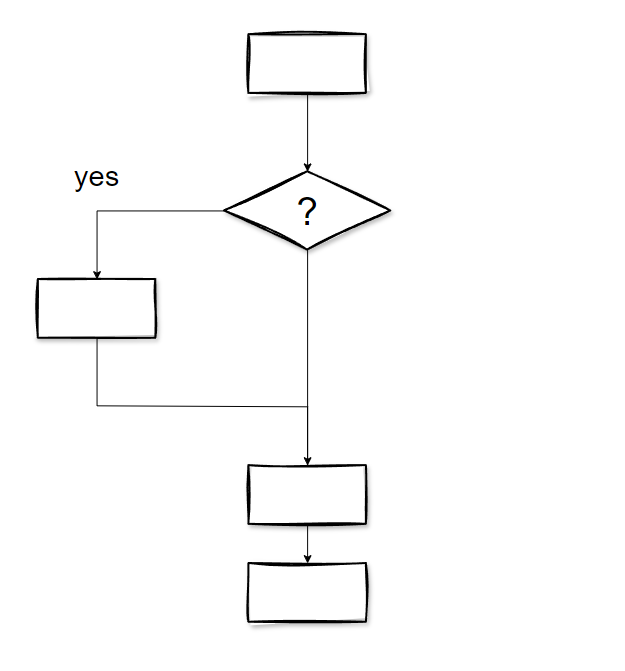
Examples
Once again, let's turn to your profiles as a source of randomness.
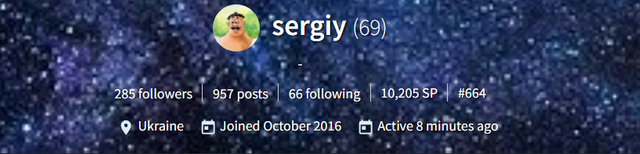
#include <iostream>
using namespace std;
int main()
{
int followers=285;
int posts= 957;
int following=66;
int steem_power=10'205;
bool posts_more_1000 = posts>1000;
if(posts_more_1000==true)
cout<<"Wow! I publish more than 1000 posts";
else
cout<<"Oh no, I’m so slow. I must be more active";
if(posts>1000)
cout<<"Wow! I publish more than 1000 posts";
else
cout<<"Oh no, I’m so slow. I must be more active";
if(followers > following)
cout<<"More users followed me than I followed";
if(following > followers)
cout<<"I followed more users than those who followed me";
return 0;
}
In this program, I created variables that store the values of parameters from my profile.
bool posts_more_1000 = posts>1000;
Here I stored the answer to the question of whether my post count exceeds 1000 in the variable posts_more_1000. The variable allows evaluating the question once and referencing the result without asking again.
Now to evaluate the number of posts, I can use this variable that already contains the answer. I only need to check the result - true or false.
if(posts_more_1000==true)
cout << "Wow! I have published more than 1000 posts!";
else
cout << "Oh no, I’m so slow. I must be more active!";
When it’s just a simple question, there’s no need to create a variable. You can ask the question directly:
if(posts>1000)
cout << "Wow! I have published more than 1000 posts!";
else
cout << "Oh no, I’m so slow. I must be more active!";
A conditional operator can be nested within another since the world is not just black and white - there are other options.
For example, you need to evaluate which of three numbers the user chose:
int human_answer;
cout<<"Make a choice (1-2-3)";
cin>>human_answer;
if(human_answer==1)
cout<<"1 - good choice";
else
But immediately writing actions for else isn’t possible, as we don’t know what the user chose. Since there are still options 2 and 3, we check them with another if inside the else:
int human_answer;
cout<<"Make a choice (1-2-3)";
cin>>human_answer;
if(human_answer==1)
cout<<"1 - good choice";
else
if(human_answer==2)
cout<<"2 - good choice";
else
and now there is nothing more to check—since there is the third, only remaining option. If neither 1 nor 2 worked, the third option remains—it's 3.
int human_answer;
cout << "Make a choice (1-2-3)";
cin >> human_answer;
if (human_answer == 1)
cout << "1 - good choice";
else
if (human_answer == 2)
cout << "2 - good choice";
else
cout << "3 - good choice";
When programming, indentation is used to help recognize which blocks of code will be executed depending on the conditions. It's also preferable to use { } to clearly mark the boundaries of the blocks (the scope of the if and else statements).
int human_answer;
cout << "Make a choice (1-2-3)";
cin >> human_answer;
if (human_answer == 1)
{
cout << "1 - good choice";
}
else
{
if (human_answer == 2)
{
cout << "2 - good choice";
}
else
{
cout << "3 - good choice";
}
}
Tasks
If it's stated that two numbers are given, you can enter them yourself:
int a = 345, b = -19;
or (this is better, but you will have to input the numbers when the program runs)
int a, b; cin >> a >> b;
- Think about the previous task, and for those reading this for the first time, I’ll remind you of its conditions. There is a number, for example, 1, and you can only add 1 to it, or you can multiply it by 2. How, using only these commands, +1 and x2, can you grow from 1 to 100? You’ve already written answers—but how did you approach it?—rhetorical question, don't answer. But what if you had to go from 1 to 13709—how would you go? This task has a very simple and easy solution. Find this idea, and you'll have it all. Find a universal and optimal solution to this problem.
- What is the purpose of Boolean variables? Why are they called that?
- What is the purpose of a conditional operator? What types exist?
- Write a program to check if you’ve subscribed to 100 users.
- Write a program to check whether you have more subscribers or more posts on steemit.com.
- Write a program to check whether you have more posts, more subscribers or more Steem Power on steemit.com.
- Given two numbers, write a program to determine whether their product or their sum is greater.
- Given two house numbers, determine if they are on the same side of the street (assuming all even numbers are on one side, and all odd numbers are on the other).
- Given a number, determine if it is true that it has more than four digits, is divisible by 7, and not divisible by 3.
- Which digit of a two-digit number is larger—the first or the second?
Each correctly completed task earns 1 point—up to 10 points in total.
Participation Rules
You can publish your work in any language, in any community, or just on your personal blog. Add the link to your work in a comment here.
To help me quickly find, review, and grade your work, leave a link in the comments under this text, and include the tag #sec20w4sergeyk in your post.
Invite two or three friends to participate.
Submit your works from Monday, September 30 to Sunday, October 6.
All your works will be reviewed by me, and I will select the top five submissions.

UA
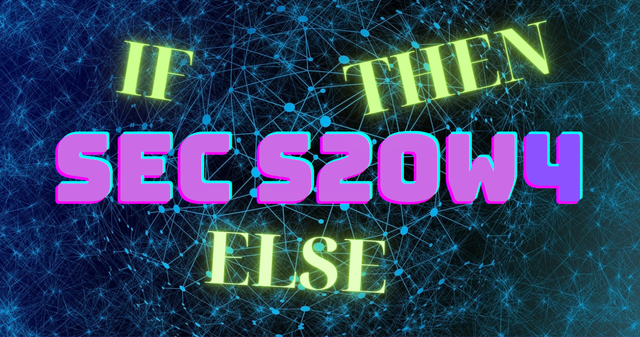
Взагалі програмування базується на двох речах умовний оператор та цикли. Умовний оператор ми вивчимо сьогодні.
Логічні змінні. Логічні операції
Спочатку трохи про логічні змінні. Логічні змінні можуть зберігати лиш два стани - 0 та 1, але вони не у всіх мовах мають суто числове значення. Здебільшого вони мають значення істини
або хиби
. Спочатку в мовах С/С++ спеціальних логічних змінних(і значень) не було, Був нуль, що розцінювався як хиба, та була 1 яка розцінювалася як істина. Навіть більше - все що не нуль - це істина, а хиба лише нуль.
В математиці, і програмуванні є логічні операції >
, <
, >=
, <=
, вони очевидні - але не очевидні операції порівняння. Коли ми хочемо порівняти значення змінних a
та b
, то ми запишем a==b
і не слід плутати з операцією присвоювання a=b
.
- операція присвоювання - це дія
a=b
, а - - операція порівняння
a==b
, це не дія а запитання - сприймайте це так.
Відповідно до перевірки на рівність є перевірка на нерівністьa!=b
Тепер прологічні вирази
- це вирази що можуть приймати значення істини або хиби, наприкладa>4
,s+3<w
,a!=78
,....
Логічні вирази можна сполучати логічними операціями -||
(логічне або) та&&
(логічне і)
Припустимо у нас є два вирази, значення їх істинності ми записали у змінніa
таb
. Яким буде результат цих виразівa||b
,a&&b
?
OR
a||b
- істинне тоді і лише тоді коли істинне одне з двох значень a
чиb
(або обидва)
a||b
- хибне лиш тоді коли вони обидва хибні
е операція - логічне або
її ще називають логічним додаванням
0+0=0
0+1=1
1+0=1
1+1=1 (так як 2 не буває, істина одна)
AND
a&&b
істинне тоді і лише тоді коли істинні обидва значення a
та b
, а хибне лиш тоді коли вони обидва хибні
цю операцію ще називають логічним множенням
00=0
01=1
10=1
11=1
NOT
Операція !
це операція заперечення, вона з істини робить хибу, а з хиби робить істину. Тобто якщо a=false
то !a
буде true
і навпаки.
це називається булевою логікою, або булевою алгеброю. Звісну о неї є свої закони, більшість з них інтуїтивні, але на початку слід уникати складних конструкцій.
От наприклад як перевірити чи число ділиться на 15?
Якщо число поділиться на 15, маємо на увазі націло, то залишок буде 0.
оператор - if then else
взагалі слово then в багатьох мовах програмування вже не пишуть, а скорочують використання до if(умова) дія при істині, та дія при хибі
це зображується такою схемою.
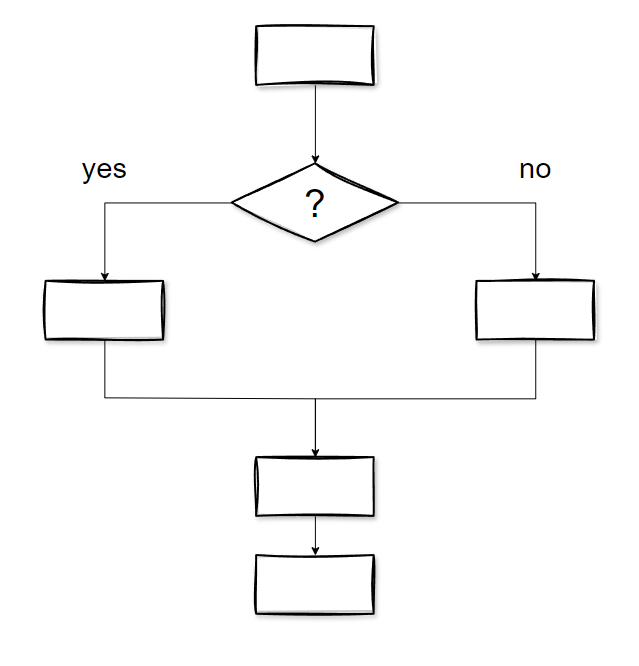
До цього визнали що команда виконується послідовно, крок за кроком. Тепер же програма може виконувати, або не виконувати певні дії.
Наприклад У Вас на рахунку
$100
, а запросили видати $200
. можна звісно видати, і це буде кредит - на балансі буде -$100
А можна це перевірити умовою.
int balance=100;
int want_withraw=200;
if(balance<withdraw)
cout<<"not much money";
else
cout<<"Take your cash";
Такий код ілюструє поведінку при знятті готівки - якщо баланс менший ніж ми хочемо зняти банкомат перевірить це умовою і прийме рішення згідно умови.
Умовний оператор може бути не повним - коли відсутня частина з else
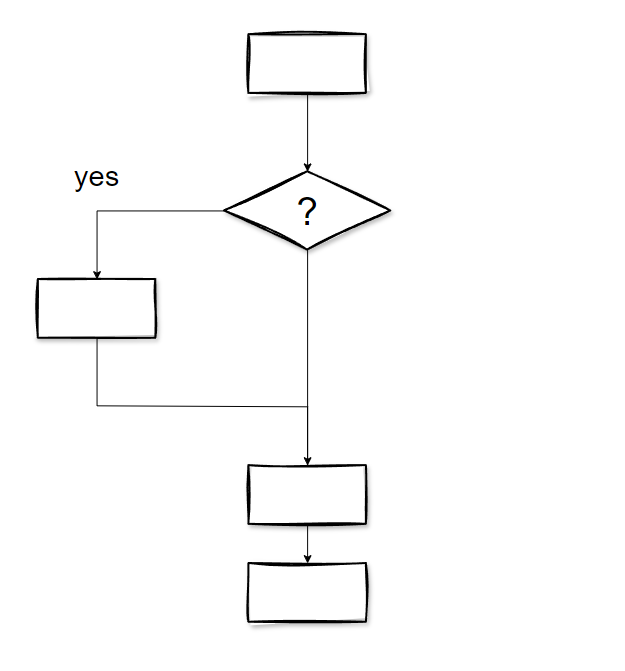
Тоді якщо умова істинна ми виконаємо певну дію, (якимось чином відреагуємо), а якщо умова хибна - то в цьому випадку ми ніяк не будемо на це реагувати.
приклади
Знову як і минулого разу, звернемося до ваших профілів, як до джерела випадковості.
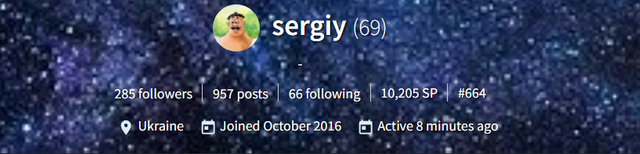
#include <iostream>
using namespace std;
int main()
{
int followers=285;
int posts= 957;
int following=66;
int steem_power=10'205;
bool posts_more_1000 = posts>1000;
if(posts_more_1000==true)
cout<<"Wow! I publish more then 1000 posts";
else
cout<<"O no, I so slowly. I must be more activests";
if(posts>1000)
cout<<"Wow! I publish more then 1000 posts";
else
cout<<"O no, I so slowly. I must be more activests";
if(followers > following)
cout<<"на мене підписалося більше користувачів ніж я";
if(following > followers)
cout<<"я підписався на більшу кількість ні підписалося на мене";
return 0;
}
В цій програмі я створив змінні що зберігатимуть значення параметрів з мого профілю.
bool posts_more_1000 = posts>1000;
тут я в змінній posts_more_1000
зберіг відповідь на запитання чи більша кількість моїх публікацій за 1000. Змінна дозволяє оцінити запитання один раз, і звертатися надалі за результатом до неї і не задавати запитання повторно.
Тепер щоб оцінити кількість опублікованих постів я можу використати цю змінну яка вже містить відповідь на дане запитання. Мені треба лиш глянути яка це відповідь - так чи ні.
if(posts_more_1000==true)
cout << "Wow! I have published more than 1000 posts!";
else
cout << "Oh no, I'm so slow. I must be more active!";
Коли це лиш одне просте запитання змінну можна і не створювати. А поставити запитання-умову відразу
if(posts>1000)
cout << "Wow! I have published more than 1000 posts!";
else
cout << "Oh no, I'm so slow. I must be more active!";
Умовний оператор може входити в середину іншого, адже світ не ділиться на чорне та біле -є й інші варіанти.
Наприклад треба оцінити яку із трьох цифр вибрав користувач;
int human_answer;
cout<<"Make a choice (1-2-3)";
cin>>human_answer
if(human_answer==1)
cout<<"1 - good choise";
else
але відразу писати команди дії для else
ми не можемо, так як в тому варіанті що лишився ми не знаємо що вибрав користувач. Так як залишилися ще варіанти 2
та 3
їх ми й перевіримо ще одним вкладеним після else оператором if:
int human_answer;
cout<<"Make a choice (1-2-3)";
cin>>human_answer
if(human_answer==1)
cout<<"1 - good choise";
else
if(human_answer==2)
cout<<"2 - good choise";
else
а тепер більш нічого перевіряти не треба - так як залишився третій, єдиний варіант. Якщо ні 1, ні 2 не спрацювало - лишився 3й варіант - це 3.
int human_answer;
cout<<"Make a choice (1-2-3)";
cin>>human_answer
if(human_answer==1)
cout<<"1 - good choise";
else
if(human_answer==2)
cout<<"2 - good choise";
else
cout<<"3 - good choise";
При програмуванні роблять відступи, вони допомагають розпізнавати блоки коду які виконуються чи ні залежно від умов.
А ще бажано розставляти { }
- вказуючи межі блоків(зону дії if та else
int human_answer;
cout<<"Make a choice (1-2-3)";
cin>>human_answer
if(human_answer==1)
{
cout<<"1 - good choise";
}
else
{
if(human_answer==2)
{
cout<<"2 - good choise";
}
else
{
cout<<"3 - good choise";
}
}
задачі
якщо сказано що дано два числа - можете самі їх ввести
int a = 345, b = -19;
або (це краще, але числа доведеться вводити під час запуску програми)
int a,b; cin>>a>>b;
- Подумайте над попередньою задачею, а для тих хто це читає вперше нагадаю її умови. Є число, наприклад
1
до нього можна лишедодавати 1
або його можнапомножити на 2
. Як з допомогою лише цих команд, тобто+1
таx2
вирости з 1 до 100. Ви вже писали відповіді - але як ви їх ходили? - rhetorical question, не відповідайте.
Але якщо треба буде дійти від 1 до 13709 - як би ви йшли? A ця задача має дуже легкий і простий розв'язок, знайдете цю ідею і все.
Найдіть універсальний оптимальний спосіб розв'язку цієї задачі. - Яке призначення булевих змінних? Чому вони так називаються?
- Яке призначення умовного оператора? Яким він буває?
- Напишіть програму для перевірки чи підписалися ви на 100 користувачів?
- Напишіть програму для перевірки чого у вас більше на steemit.com підписників чи опубліковано постів?
- Напишіть програму для перевірки чого у вас більше на steemit.com опубліковано постів, підписників чи Steem Power?
- Дано два числа, напишіть програму яка визначить що більше добуток цих двох чисел чи сума.
- Дано два номери будинків. Вияснити чи знаходяться вони на одній стороні вулиці. (сподіваюся у всіх по один бік вулиці парні номери, а по інший бік непарні)
- Дано число вияснити чи правда що воно більше ніж з чотирьох цифр, чи ділиться на 7 і не ділиться на 3.
- Яка цифра у двозначного числа більша перша чи друга?
Всі вірно зроблені завдання дають 1 бал - разом 10.
Правила проведення
Публікувати можна на будь-якій мові, в будь якій спільноті чи просто у власному блозі, посилання на вашу роботу додайте сюди коментарем
Щоб я швидко знайшов, перевірив та оцінив ваші роботи залиште посилання в коментарі під цим текстом а в роботі поставите тег #sec20w4sergeyk
Порекомендуйте прийняти участь своїм двом-трьом друзям.
Роботи слід опублікувати з Monday 30 Sep 24 to Sunday 6 Oct 24
Всі Ваші роботи будуть мною оцінені та відібрані п'ять кращих робіт
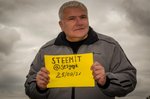
About me
Файно, поки ще більше-менш просте, то можна троки покумекать))
Як правильно виконати шосте або п'яте завдання?))
дякую що помітили....виправив завдання 6 + ще й на англійську переклад зробив, має бути 99% таким же))
I suggest you to add English translation in your post
Thank you for the suggestion! I have just translated the post into English.
Thank you so much for taking a quick action 🫂
Here is my entry: https://steemit.com/sec20w4sergeyk/@mohammadfaisal/sec-s20w4-decision-making-in-programming-if-then-else
Your post has been rewarded by the Seven Team.
Support partner witnesses
We are the hope!
https://steemit.com/hive-145157/@luxalok/sec-20w4-or-or-bulkotlivi-operatori-umovni-ta-logichni
My entry:
https://steemit.com/sec20w4sergeyk/@kouba01/sec-s20w4-decision-making-in-programming-if-then-else
Congratulations, your post has been upvoted by @scilwa, which is a curating account for @R2cornell's Discord Community. We can also be found on our hive community & peakd as well as on my Discord Server
Felicitaciones, su publication ha sido votado por @scilwa. También puedo ser encontrado en nuestra comunidad de colmena y Peakd así como en mi servidor de discordia
My entry
https://steemit.com/sec20w4sergeyk/@daprado1999/sec-s20w4-decision-making-in-programming-if-then-else
Check out my entry: SEC S20W4: Decision Making in Programming – If, Then, Else.
Also, the post is shared on Twitter: Twitter Link.
Hello sir, @sergeyk, please this is my entry;
https://steemit.com/sec20w4sergeyk/@iddy/sec-s20w4-decision-making-in-programming-if-then-else