"Learning ANSI C" - Most popular high-level computer programming language [Episode #02]
Hello guys,
Welcome to new episode of "Learning ANSI C". In the previous episode we discussed about "Introductory part of C Language". Today we'll discuss on "Structure of Program in C Language". So, let's start-
Structure of Program in C Language
Here I show you a simple program written in C language. In this program it calculates the profit from Investment & shows the result on the computer screen:
/*A sample program written in C to calculate profit from Investment*/
/* Programmed by @royalmacro */
#include <stdio.h>
#define ROI 7.88 //Here Return On Interest Value is defined as Constant
int period=5; //Global variable declaration
//main function
void main()
{
float principal=10569.67; //Local variables
float profit;
profit=calculate_profit(principal); //Calculate profit by calling user-defined function
printf("Principal:$%f USD : Period:%d Years : ROI:%f% : PROFIT = $%f USD",principal,period,ROI,profit); //Shows result on Computer screen
}
//User-defined function
calculate_profit(x)
float x;
{
float p;
p=((x*ROI)/100)*period; //calculate profit
return(p); // return value
}
Output on Computer Screen:
Principal:$10569.67 USD : Period:5 Years : ROI:7.880000% : PROFIT = $4164.44998 USD
Now please, close look at the above code. You see a well structure in that program.
Basic Structure of C Program
- Documentation Portion
- Link or, Header File Portion
- Definition Portion
- Global Declaration Portion
- main() Function Portion
- Sub-program Portion
Documentation Portion
Every program starts with "Documentation Section". It consists of a set of comment lines which describes the name of the program, purpose, programmer's name & any other program related info.
/*A sample program written in C to calculate profit from Investment*/
/* Programmed by @royalmacro */
In the above program this section is called "Documentation Portion".
You see that I write text in Documentation Section within /* & */ .
Every texts start with /* (slash asterix mark) & ends with */ (asterix slash mark). To write a comment or text which will not be complied must be written in that format. Otherwise, program will not be complied correctly & will show fatal errors.
There are two types of commenting --
(i) Single-line Commenting
(ii) Multiple-line Commenting
(i) Single-line Commenting:
This type of comment must starts with double slash(//) or within /* (slash asterix mark) & */ (asterix slash mark).
#define ROI 7.88 //Here Return On Interest Value is defined as Constant
In the example program you see the use of single line comment.
You can write this comment in another format --
#define ROI 7.88 /*Here Return On Interest Value is defined as Constant*/
(ii) Multiple-line Commenting:
This type of comment must starts with /* (slash asterix mark) & ends with */ (asterix slash mark).
/*A sample program written in C to calculate profit from Investment.
Programmed by @royalmacro */
So, we learnt that "double slash(//)" is only used for single-line comment but "/* (slash asterix mark) & */ (asterix slash mark)" is used both for single-line & multi-line comment.
Link or, Header File Portion
We previously learnt that C program is basically a set of functions. When we call a function C compiler search the definition of that function & executes commands from C library of "Functions". For doing this compiler needs to link functions from C library. The "Link/Header File Section" do that job.
#include <stdio.h>
In the above program this section is called "Link or, Header File Portion".
"#include" is a preprocessor directive that specifies the files to be included in C program. "stdio.h" is a header file in which all standard input/output functions definitions are stored. In the above program we used printf() function, this function's definition is stored in "stdio.h" header file. Without linking correct header file the compiler will be unable to compile the codes.
To link a header file we write like this in Link/Header Section-
#include <stdio.h>
or,
#include "stdio.h"
We must use hash(#) before "include" then write the header file with/without file path. Every built-in header file is located in default root folder, so, we do not need include the header file with path. But, if we develop custom header file and want to include in Link Section then we must cite the full path like this--
#include <C:\TURBOC3\INCLUDE\AI.H>
Definition Portion
In the "Definition Section" it defines any type of symbolic constants. "#define" defines a macro substitution.
#define ROI 7.88 //Here Return On Interest Value is defined as Constant
In the above program we define ROI as a constant and assign a value of 7.88. We can use ROI constant in any part of the program globally. In the program there is no chance to change the value of ROI.
In the above program we used ROI in user-defined function.
p=((x*ROI)/100)*period;
When program executed it assigns the value of ROI to 7.88 as we defined first it in the "Definition Section". If we try to assign new value of ROI in main function or user-defined function then compiler shows fatal error. So, we can not change the value if we define it as a constant in "Definition Section".
Global Declaration Portion
In C program we can use two different types of variables - Local & Global. Local variables are declared in one & can not be used outside of that function. But, Global variables are declared in "Global Declaration Portion" & can be used in any functions of the program.
Global variable
int period=5; //Global variable declaration
Local variable
float principal=10569.67; //Local variables
Look period is declared as global variable in Global Declaration Section & principal variable is locally declared in main() function. You can use principal variable within main() function. But, look, we used period variable outside main().
profit variable can only be used in main()
profit=calculate_profit(principal); //Calculate profit by calling user-defined function
period variable can be used in both main() & user-defined function
p=((x*ROI)/100)*period; //calculate profit
main() Function Portion
C compiler starts to compile from here. Every C program must have one main() function. Contents of main() function must be appear between opening & closing braces. The C program execution starts at the opening brace "{" & ends at the closing brace "}".
It has two sections--
(i) Variable declaration section
(ii) Execution Section
void main()
{
// Variable declaration section
int a,b;
int c;
//Execution Section
a=12;
b=45;
c=a+b;
printf("%d",c);
}
Every function must have two parentheses, begin parenthesis "(" & end parenthesis ")". Every statement in the variable declaration part & execution part ends with a semicolon ";". And mind it that C is a case sensitive language. So, if you write printf() function as Printf() then it'll be not complied correctly. All functions & variable declarations are written in lower-case form.
//main function section
void main()
{ //opening brace
// Variable declaration section
float principal=10569.67; //Local variables
float profit;
// Execution Section
profit=calculate_profit(principal); //Calculate profit by calling user-defined function
printf("Principal:$%f USD : Period:%d Years : ROI:%f% : PROFIT = $%f USD",principal,period,ROI,profit); //Shows result on Computer screen
} //closing brace
Sub-program Portion
The sub-program portion is a set of all user-defined functions which are called in the main() function. The example of sub-program-
//main function
void main()
{
float principal=10569.67; //Local variables
float profit;
profit=calculate_profit(principal); //Calculate profit by calling user-defined function
printf("Principal:$%f USD : Period:%d Years : ROI:%f% : PROFIT = $%f USD",principal,period,ROI,profit); //Shows result on Computer screen
}
//User-defined function
calculate_profit(x)
float x;
{
float p;
p=((x*ROI)/100)*period; //calculate profit
return(p); // return value
}
Previous Episodes:
[Episode-01]
[To be continued....]
follow me on steemit AND resteem it
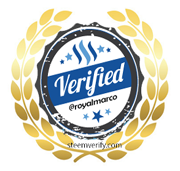
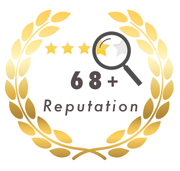
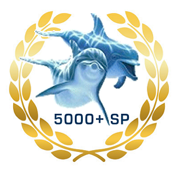
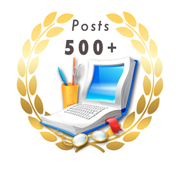
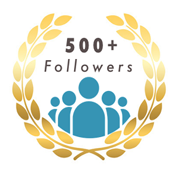
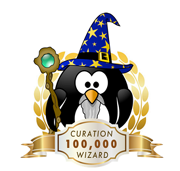
>>Thanks to @elyaque for designing my badges :)<<
MY STATS
REPUTATION SCORE : 72.30 | TOTAL FOLLOWERS : 3118
TOTAL BLOG POSTS : 1923 | TOTAL LIKES : 105864
TOTAL EARNINGS : $32406.94 SBD
Wow this looks like something way over my head. I don't like to spend much time on the computer, so this is probably not for me. But it looks like you are sharing some great information to many who can use it so that is great. Just was poking around the platform and stumbled up @photoman and that brought me over to your page. Anyway I've been on Steemit since 2017, not as long as you, but it's always a pleasure to introduce myself to another steemit veteran. Maybe you know @firepower he's from, and I got the pleasure to meet him In Krakow Poland at steemfest. Very good guy.
So I've been traveling for 18 years and I was going to do my first adventure in India, then covid hit while I was motorbiking through Vietnam. I got on one of the last planes to Thailand back in March 2020 and have stayed here since.
So I don't much about India but I do know you guys have some great food and spices! The people I've met from your country have always been very nice. Anyway, just wanted to stop by and drop a note, and say I think it's great that you have been helping people with the programming and much more here on Steemit. Keep up the great work!
From Koh Phangan Thailand,
-Dan "World Travel Pro!"
Thank you @world-travel-pro for elaborating your thinking very nicely. Actually C programming is not very hard, and anyone can learn it easily. However, after this pandemic situation is over please visit our country again; I'll be honored :)
Indeed. I'll certainly have to come to India once things settle down. Maybe I can even participate in a little Steemit Meet-up India style. That would be cool. Until them I'll be in touch. Have a great day!
good gentleman thanks for share your another episode.
thanks :)
Thank you for sharing. I hope it will be helpful for many people who wants to learn C @royalmacro
Although it's a basic tutorial, but, I hope it'll help novice programmer
Good to see your post again @royalmacro
yeah, thanks :)
Excellent! @royalmacro keep posting.
You're most welcome :D
best explanation,