SLC S22 Week2 || The Object Approach In JAVA
Greetings, Earthling! Are you ready to debug the matrix?
Below you can find the homework for this weeks Java class by @kouba01, you can find more details here SLC S22 Week2 || The Object Approach In JAVA.
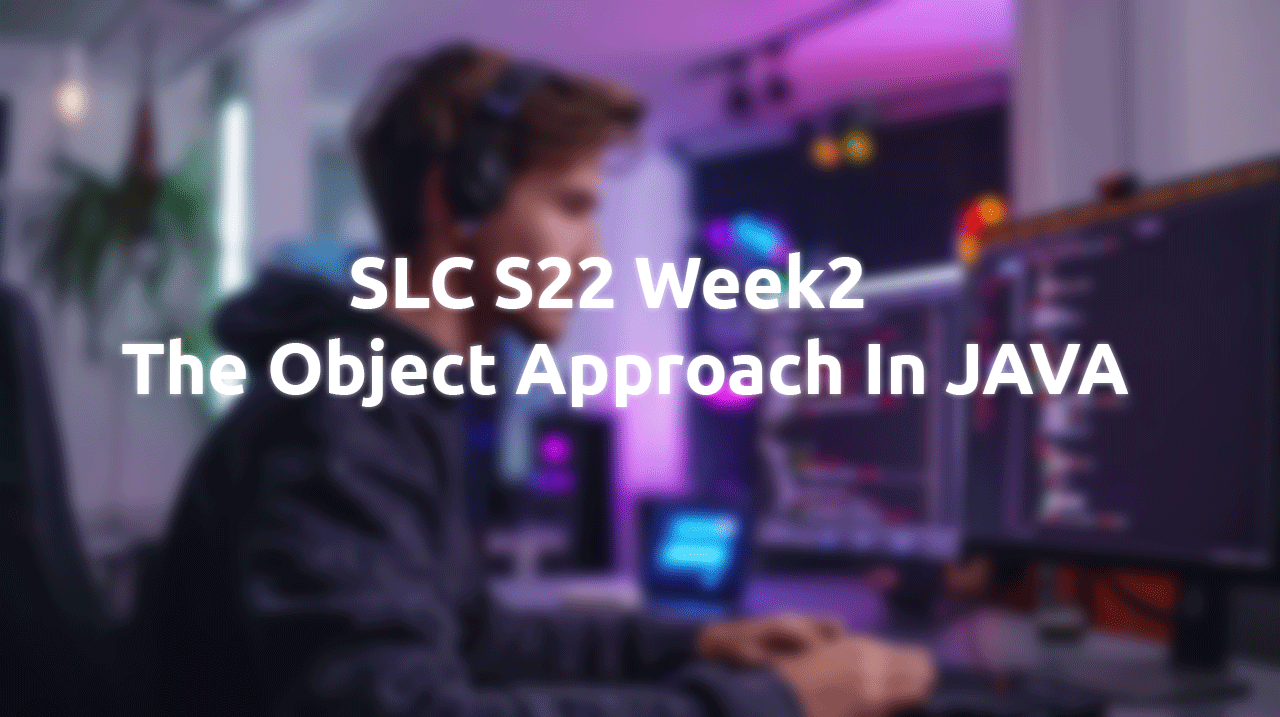
Image taken from Pixabay

Which of the following statements about Java classes and objects is true?
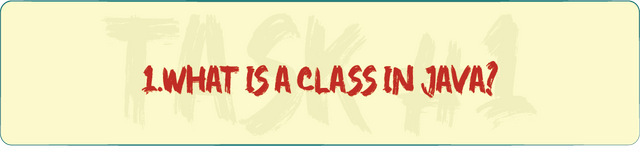
- (A) A blueprint for creating objects.
- (B) A specific instance of an object.
- (C) A library of pre-written code.
- (D) A type of variable used to store data.
Answer
- (A) A blueprint for creating objects.
A class is like a blueprint or template that describes how an object needs to be created. Think of it as a recipe to create objects.
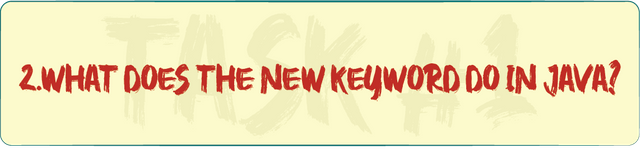
- (A) Declares a new variable.
- (B) Creates a new object of a class.
- (C) Adds a new method to a class.
- (D) Initializes a static variable.
Answer
- (B) Creates a new object of a class.
The new
term in Java is used to create a new instance of a class, by this creating a new object.
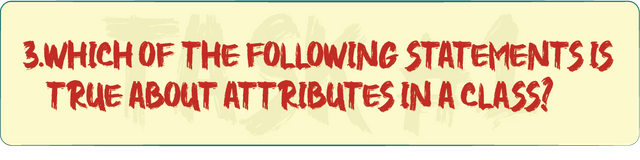
- (A) Attributes are also known as methods.
- (B) Attributes must always be public.
- (C) Attributes store data for an object.
- (D) Attributes are optional in a class.
Answer
- (C) Attributes store data for an object.
Each class/object has it's own attributes used to store data. Each instance of a class can have it's own different values for the attributes.
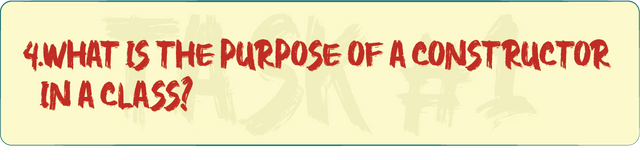
- (A) To define the behavior of an object.
- (B) To initialize the fields of a class.
- (C) To execute code when a method is called.
- (D) To create a copy of an existing object.
Answer
- (B) To initialize the fields of a class.
In Java the constructor is a "special" method that is called when the object is being created, it is used to initialize the fields. A constructor can have parameters and usually it comes with the same name as the class where it is being used.

Write a program that demonstrates the creation and use of a class by including attributes, methods, and object instantiation. Specifically, create - a Laptop class with attributes brand (a string) and price (a double).
Implement a constructor to initialize these attributes and a method displayDetails() to print the brand and price of the laptop.
In the main method, create two instances of the Laptop class with different values for the attributes and call the displayDetails() method for each object to produce and display the corresponding output.
We start this task with the creation of the Laptop class, we've learned the process of creating a new class in last week's course. Once we have the class created we start with the first point of this task.
Declaring the attributes, brand
as a String and price
as a Double:
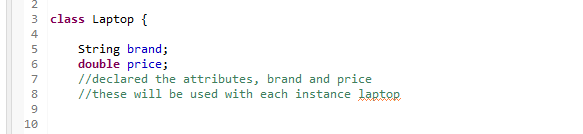
The second point of the task asks for a constructor where we initialize the attributes and a method displayDetails()
where we show the brand and price of the laptop. We have laptop1, this.
will pass the values for laptop1, when we create laptop2, it will pass the values to laptop2 and so on for each created object.
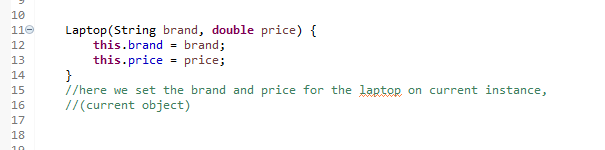
We named the constructor after the class name, Laptop
and we use the this.
prefix to tell the compiler that the brand
and price
is for this current laptop when it's created.
Let's see how the method displayDetails()
would look like, in this case the method is used just to display details about the current selected object so all we have to do is to print the values of brand
and price
. The method used in combination with the object will pinpoint to the object's values like laptop1.displayDetails()
will show the brand
and price
values only for laptop1, laptop2.displayDetails()
for laptop2 and so on.
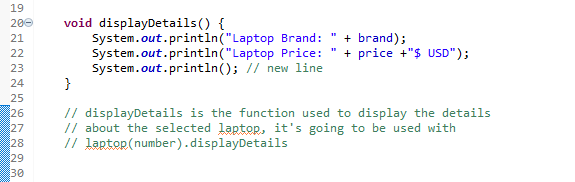
Also added an empty println
for a new line between objects, otherwise it would be clogged.
All that is left to do is to create the objects in the main
and display them after, we are going to use the constructor to create the objects and with the term new
we are going to give the values.
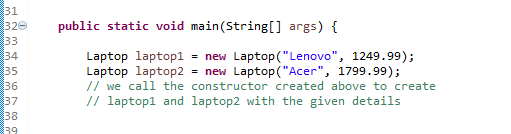
Now we have two laptops, let's show the details for both.

Also below I will leave you a small GIF example with the code being run, you can see in the console how the laptop1
and laptop2
are being printed in the Console.
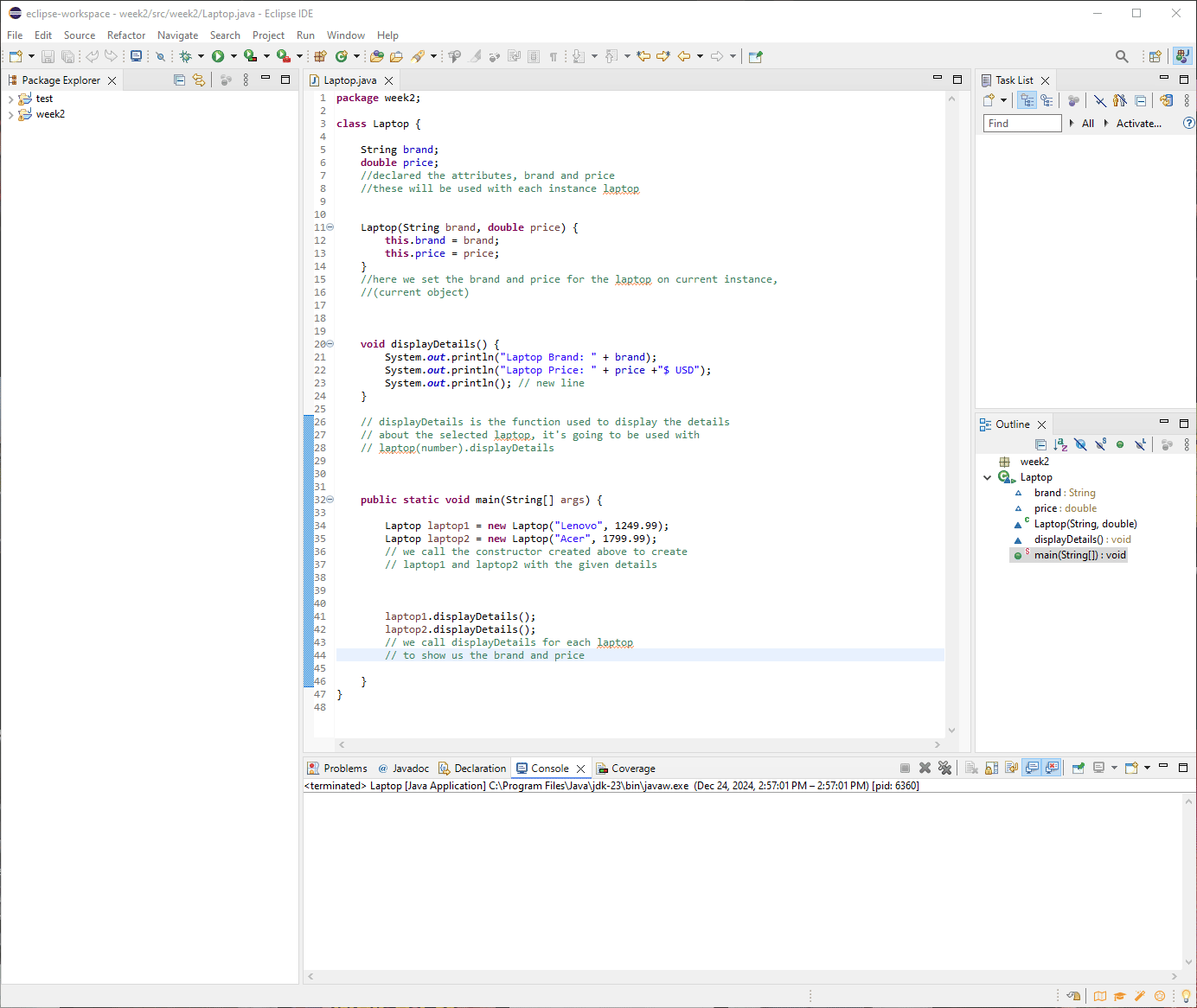

Write a program that demonstrates the creation of a Movie class, its attributes, and methods, along with managing multiple objects in an array. Define the Movie class with the attributes title (a string) and rating (a double).
Implement a constructor to initialize these attributes and a method displayInfo() to print the title and rating of the movie.
In the main method, create an array to store three Movie objects, populate the array with details for each movie, and call the displayInfo() method for each object to display their respective details.
Same as we did for the previous task, we declare the attributes String
and Double
for title and rating, after that we create the constructor Movie
and the displayInfo Method.
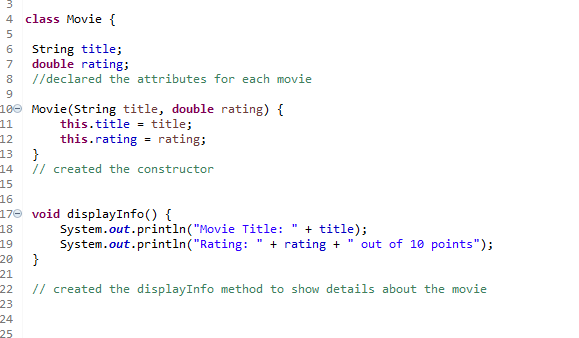
Now for the array part, we first have to create the array with a size of 3 to fit the requested movies, Movie[] movies = new Movie[3];
, and for each position of the array we are going to create a movie with it's title and rating.
Example:
movies[1] = new Movie("Shrek", 8.0);
-> where movies[1] represents the position in the array where we are creating this movie.
We can do this for all the positions of the array, once we are done we need to show the details (title+rating) for each movie. With a for loop we loop through the array of movies and for each position we show the title and it's rating, like this:
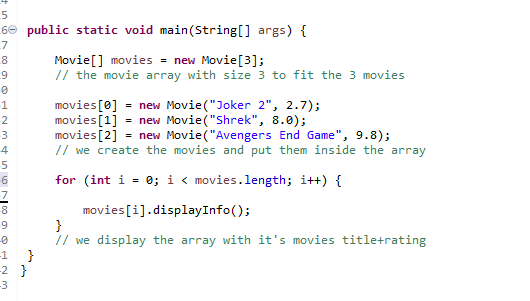
Let's also see a GIF with the code being run, you can see the results in the console.
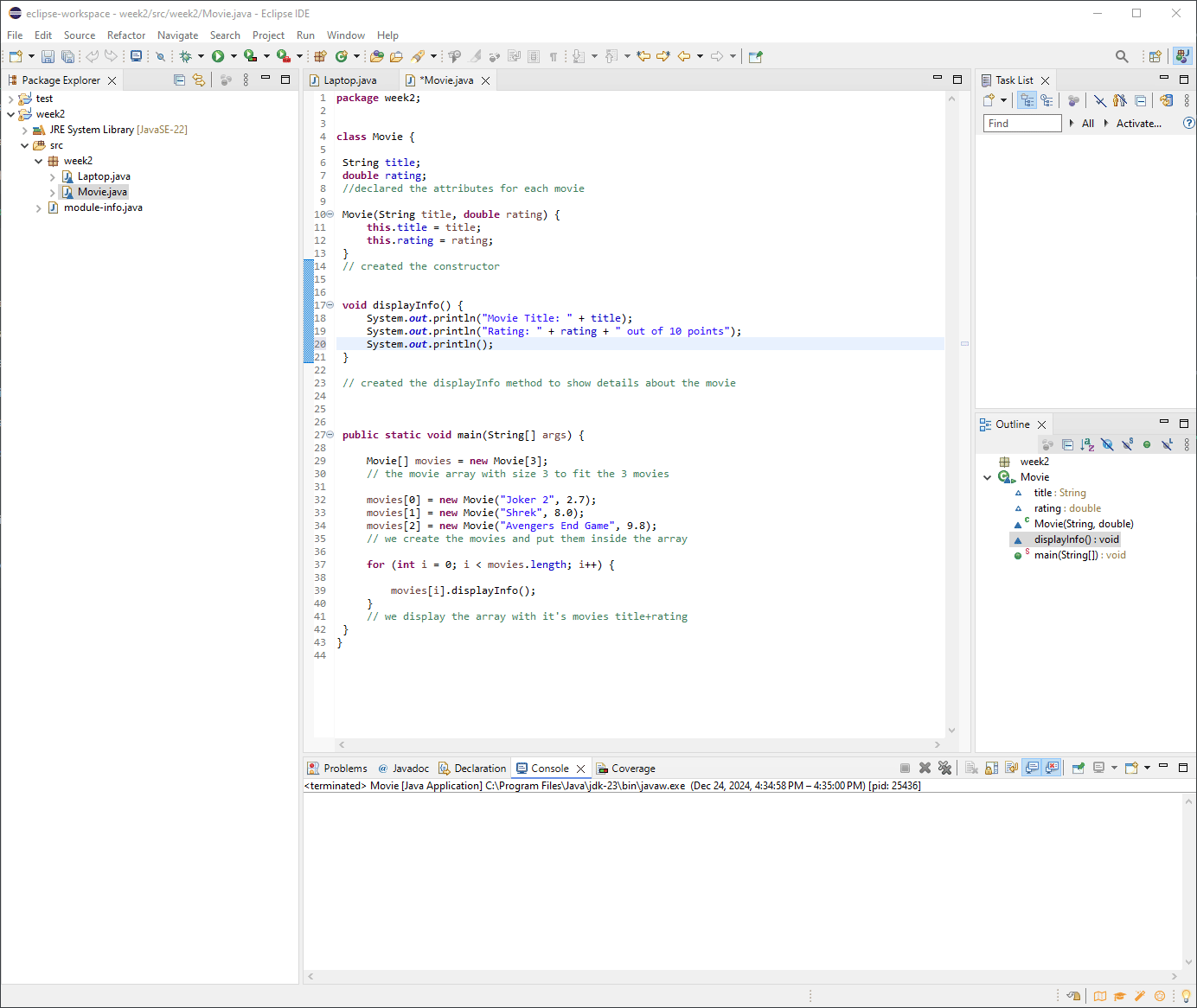

Write a program that demonstrates adding methods with calculations by creating a Product class. The class should include the - attributes name (a string), price (a double), and quantity (an integer).
Implement a constructor to initialize these attributes and a method calculateTotalPrice() to compute the total price as the product of price and quantity. Additionally, create a method displayProduct() to print the product's details, including the total price.
In the main method, create an instance of the Product class, and call the displayProduct() method to output the product's details and its total price.
From what I see @kouba01 started with something easier and each task he added a new thing to increase difficulty and add a new thing to the challenge.
Here you can see the attributes and the constructor, nothing different from the tasks above (only if you count an extra attribute).
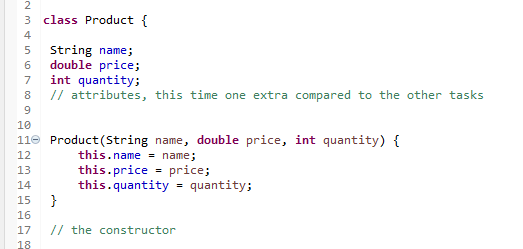
For the total price we need a method that multiplies the quantity with the price, it's going to be double and return the multiplying between the two directly.
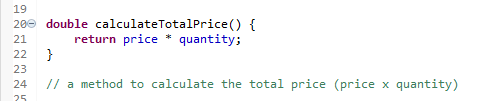
Now for the displayProduct()
the only new thing we have from the other tasks is that we have to print call the calculateTotalPrice()
method to show total price.
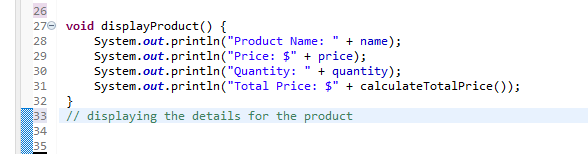
Here is the new/different thing System.out.println("Total Price: $" + calculateTotalPrice());
instead of havin a + variable
we have + function
.
In the end we create the product and display the details, like this:
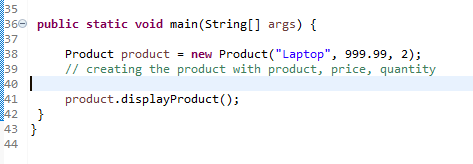
Let's see it live in a GIF:
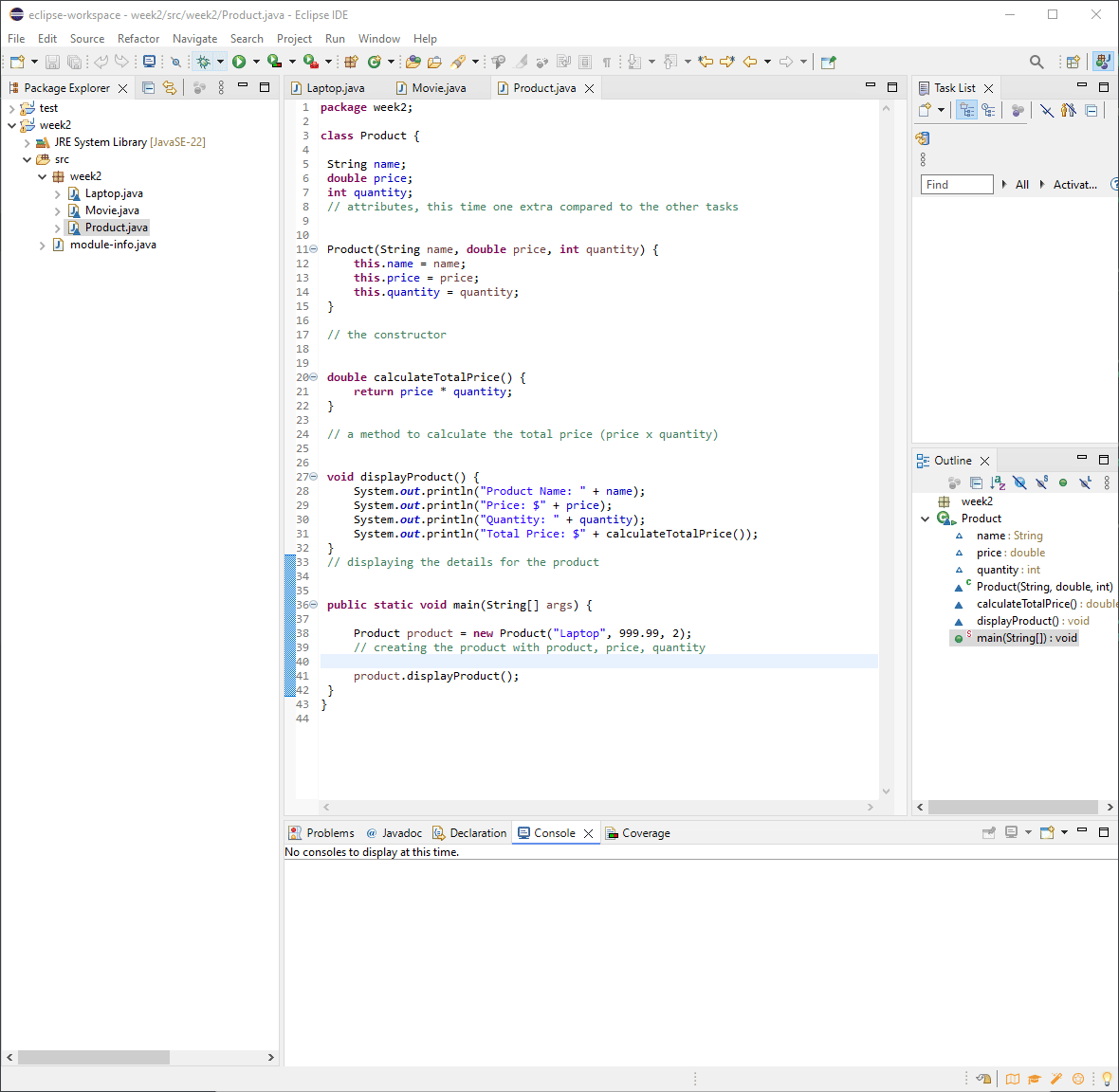

Write a program that manages student records by creating a Student class. The class should have the attributes id (an integer), name (a string), and marks (an array of integers).
Implement a constructor to initialize these attributes and a method calculateAverage() to compute and return the average of the marks. Additionally, create a method displayDetails() to print the student's details, including their average marks.
In the main method, create an array of three Student objects, populate the array with details for each student, and call the displayDetails() method for each object to display their respective information, including their average marks.
I will skip describing the attributes and constructor, it's the same process as described in the tasks above, I will only show a screenshot with them.
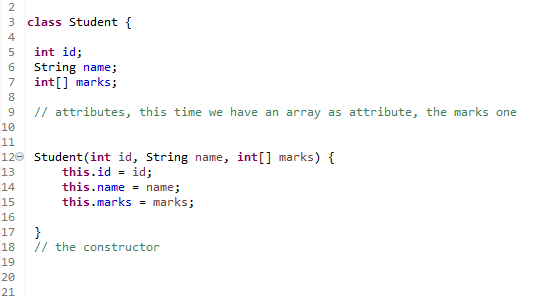
The average of the marks needs to be calculated by the sum of the marks over the number of marks, so we sum up all the marks we have in the array using a for loop and a sum variable, once we have the sum we return the sum divided to the arrays length.
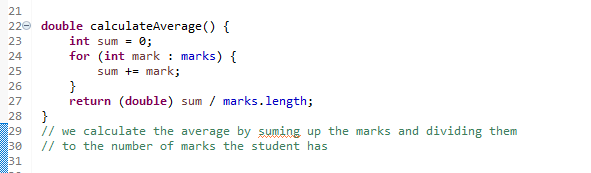
For the display method, we display the name like any other display we did so far, but for the marks because we have an array we need a loop to help us print each mark value.
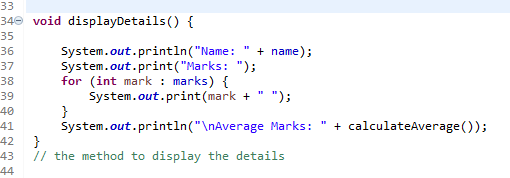
In the main
I created the students array, created 3 students with the proper details and after that I showed them with everything related to them.
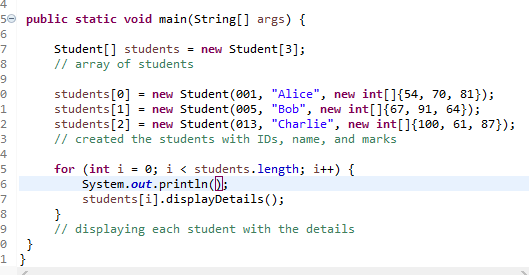
Now let's see it run in the GIF below:
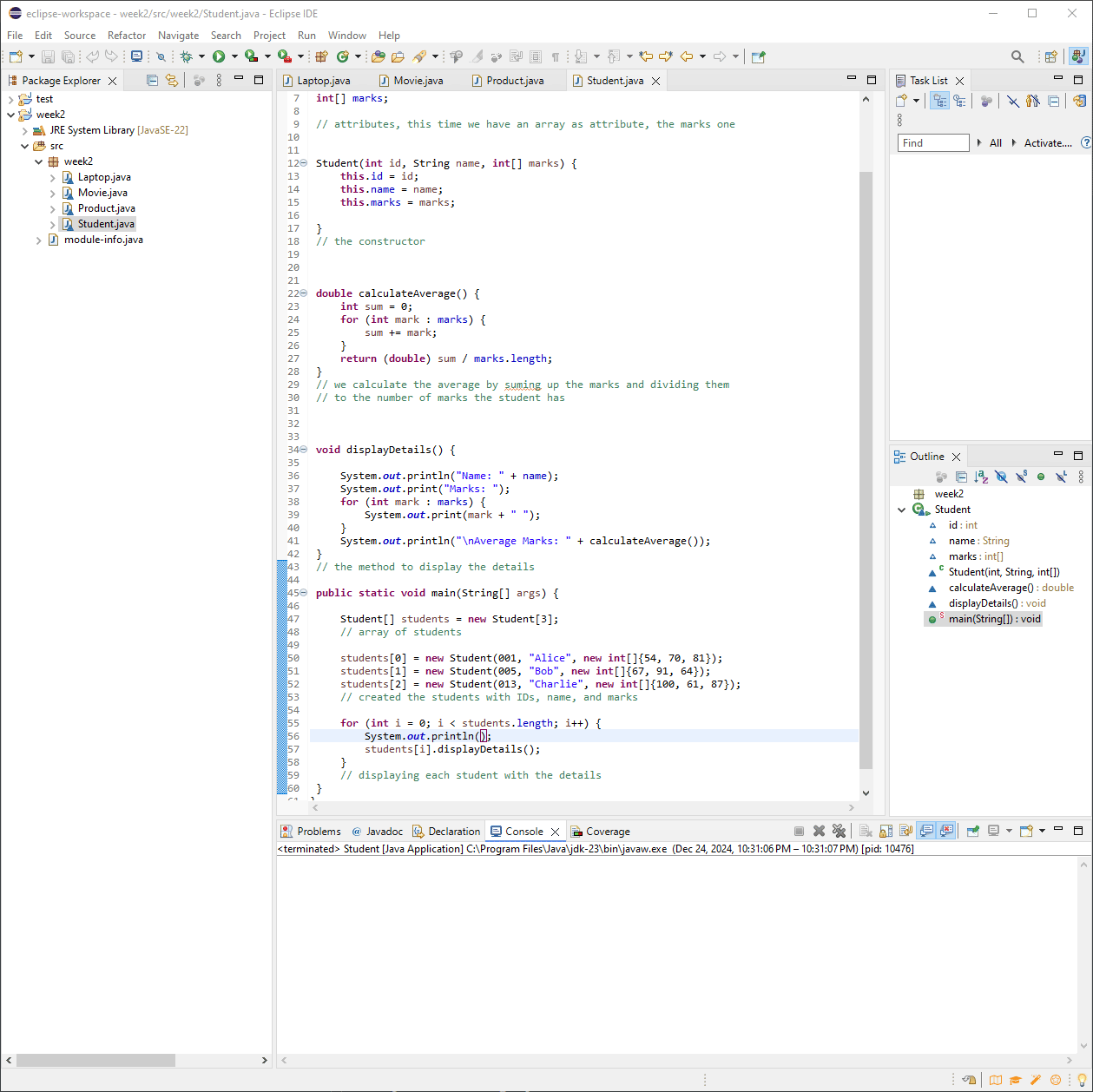
After creating everything and writing here I realized I should have made the average show only 2 decimals, so I did it now by adding these:
import java.text.DecimalFormat;
DecimalFormat df = new DecimalFormat("#.00");
to format it to 2 decimals
System.out.println("\nAverage Marks: " + df.format(calculateAverage()));
to show the average with only 2 decimals
The final result looks like this now:
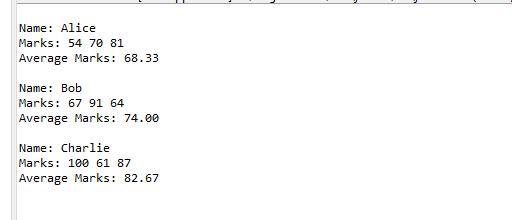

After all the tasks done above this feels like the final boss, difficulty increased. I will take each class one by one and explain what I've done, in the end I will show you the application in a GIF with all the options working.
For the Book class, I've defined the required attributes, created the constructor:
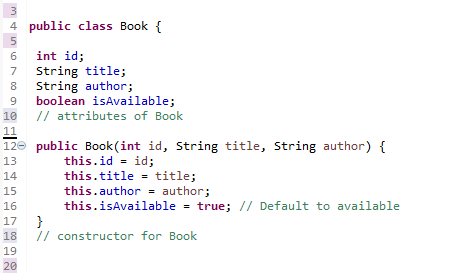
After this we need two methods one that allows us to borrow a book and one to return the book, we can do this with the boolean isAvailable
. If the variable is true, we can borrow the book otherwise it's false so someone has it already. Same for the return book method, if isAvailable is false, we can return it and the variable becomes true.
Everything should looks like this:
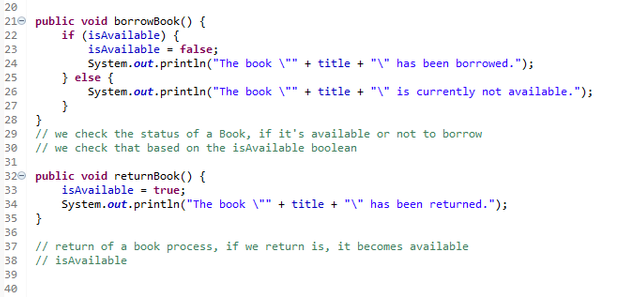
All that needs to be added is a method for the details:
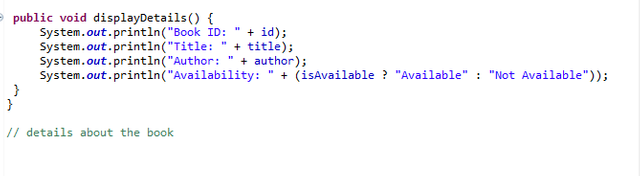
To keep everything tidy I've made the last condition with a short if to simplify things.
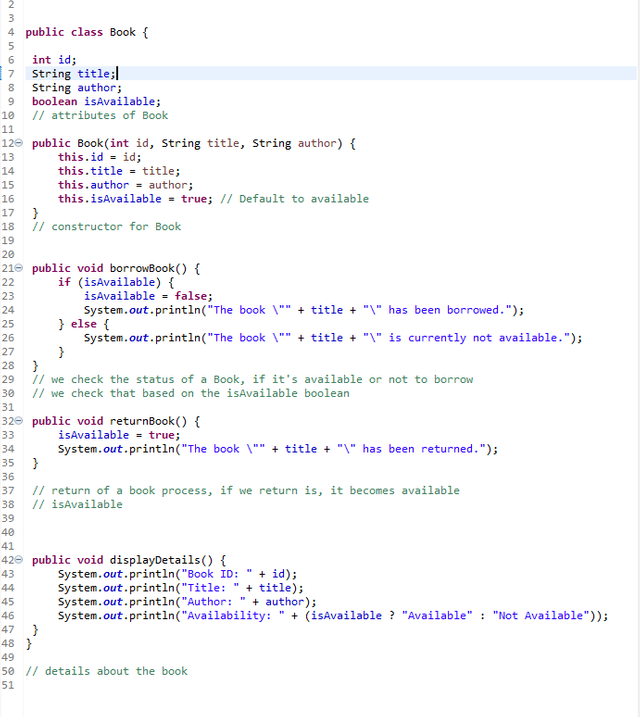
Let's move to the next class, Library, after creating the constructor and adding the attributes I wend to create the needed methods.
We need a method to add a book, once the book is added we let the user know that the title is in the library now.

We need to be able to display all the books with the details, gonna use --------------------
to split them to be visible.
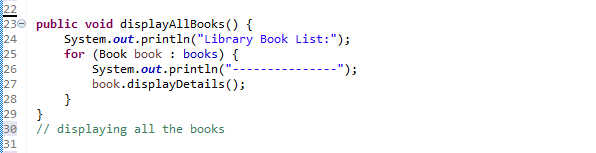
For the searching by title we are using a boolean to stop the search and the loop once we found the book, if found we display the details otherwise found remains false and we let the user know we can't find the title. Also using IgnoreCase to make searching easier, this way we don't have to make sure we write lowerscase or uppercase letters.
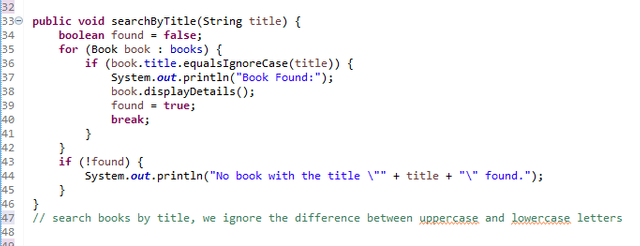
For the borrow/return book we loop through books and based on the id of the book we can borrow/return one, if it can't be found we let the user know via message.
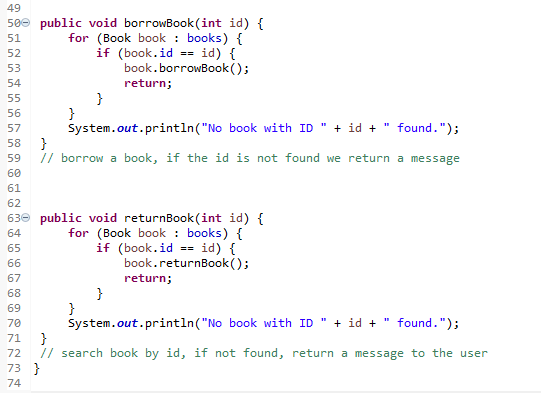
Moving to the last class, LibraryManagementSystem, here the magic takes place, we put everything together and run the library.
Here we need to get input from the user, show him the options that can be used in the library and based on his input we call methods. From 1 to 5 there are options to be picked, anything else falls on the default case where the user is alerted by an invalid choice.
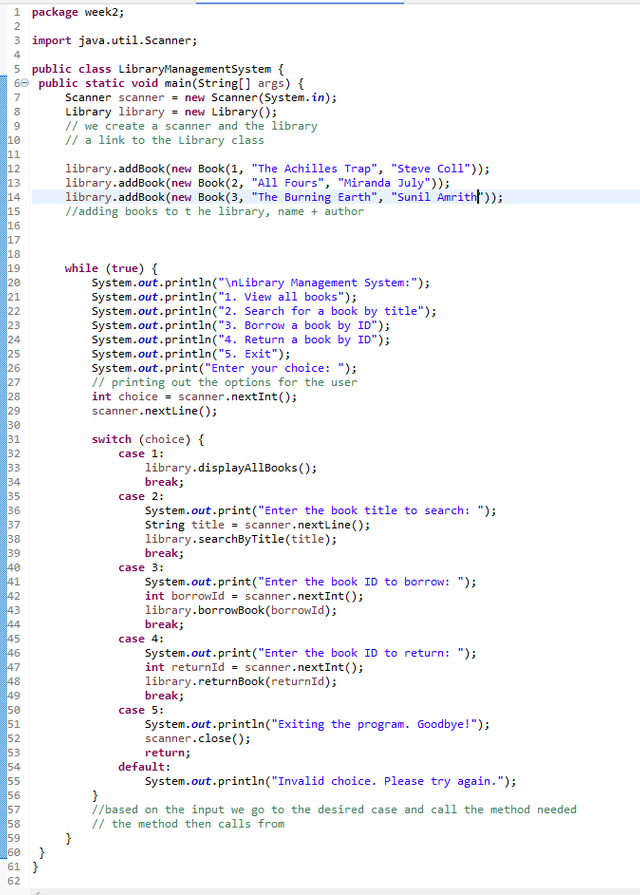
Let's check it live in action (30s GIF with all the options, expanded console to be visible)
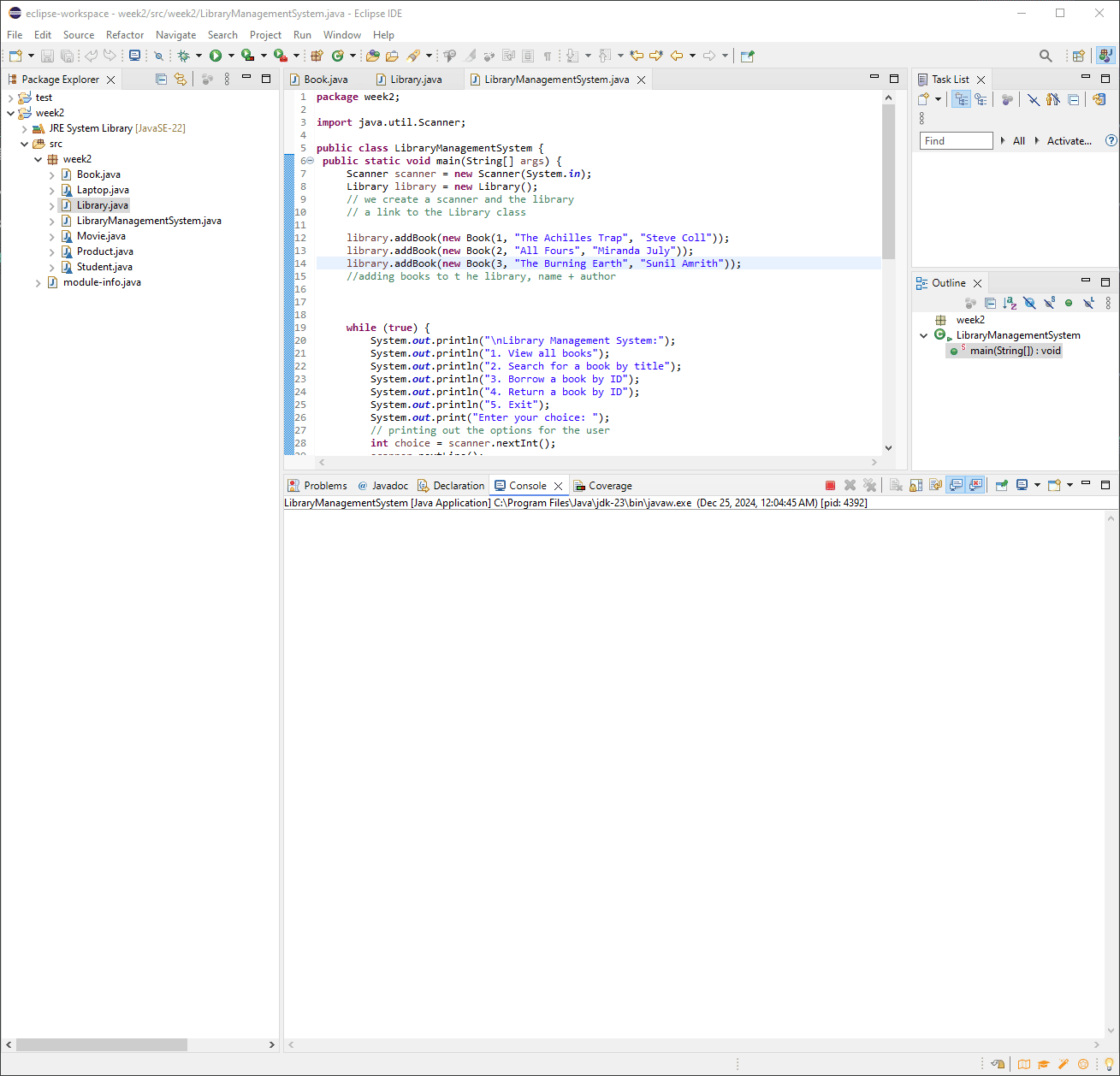
That was it for this week's Java assignment, in the end I'd like to invite @r0ssi, @titans and @mojociocio to give it a try.
Thank you again @kouba01 for this class, can't wait for the next week's one.
Until next time I am wishing you a great day and Happy Holidays!
0.00 SBD,
0.03 STEEM,
0.03 SP
Shared on X:
https://x.com/Ady_Was_Here/status/1871689632980836728