Babby's First Decentralized App: A Tutorial by a n00b for n00bs, Part 1
Want to try your hand at building a simple (and decentralized) app on the Ethereum blockchain but too much of a n00b to know where to even begin?
If this sounds even a little bit like you, then you've come to the right place!
Welcome to...
What are we making?
We're making a decentralized election app (or Dapp) by using smart contract(s) on the Ethereum Blockchain!What's a Smart Contract?
From Wikipedia - "A smart contract is a computer protocol intended to digitally facilitate, verify, or enforce the negotiation or performance of a contract. Smart contracts allow the performance of credible transactions without third parties. These transactions are trackable and irreversible."What's a Blockchain?
In a previous post I linked to a short video that explains what the blockchain is and what it does. You can also watch it below.Why can't we just build a centralized web app, huh?
Nonono... Data, rules, and such can be changed and tampered with on a centralized app. Remember the blockchain video? Everything will be verified and confirmed by the network. We want our app to be:- Decentralized
- Made so that it's one vote per person.
- Made so that the votes can't be altered.
- Made so that whoever has the most votes, wins (obvi).
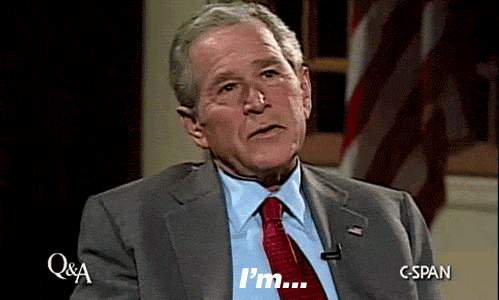
What do we need?
- Solidity - A programming language for writing Smart Contracts
- Sublime Text will be our text editor of choice.
- A terminal (I use GitBash - you can get it here. Install and fire it up).
- Node Package Manager (NPM). You might already have it, let's check first by going to GitBash and typing
node -v
and hitting Enter. If you see a version number that looks something likev.8.2
come up, then you already have it. - If not then type
brew install node
and hit Enter.
Feeling less fancy? You can download Node directly from here. - Truffle Framework - A toolset that lets us write, test, and deploy smart contracts to the blockchain in Solidity.
In your GitBash terminal typenpm install -g truffle
and hit Enter. - Ganache - A delicious sounding local Ethereum blockchain we can use to test our little project.
- Metamask for Chrome We need a plug-in to be able to use our Ethereum network.
- Go ahead and add the plug-in to your Chrome browser. Once added, you'll want to make sure it's enabled by clicking the little fox head in the upper right corner of your Chrome broswer.
- Package Control for Solidity syntax highlighting .
For some reason I got stuck for a bit trying to install Package Control, so I found that the video below helped get me through it (even though I did the exact same thing before I found the video. Go figure).
- Search under "install package" as shown in the video and the search for "ethereum" and select "Ethereum Solidity language syntax for SublimeText".
- Repeat the steps to get "EthereumSoliditySnippets" as well.
Okay I installed everything. Now what?
Awesome! The first thing we want to do is open Ganache.
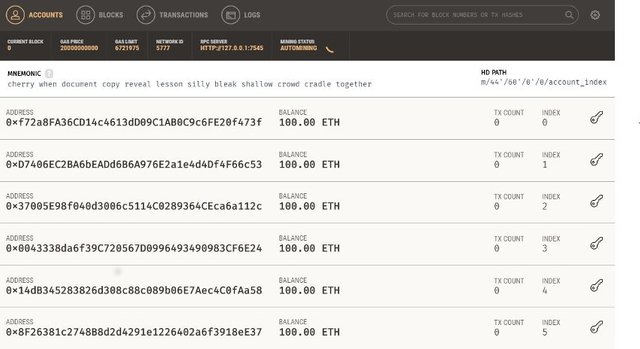
Looking at Ganache you'll see Etherium wallet addresses with 100 Ether in each.
Before you jump up and down and cover me in smooches, the Ether is fake and not worth anything.
This Ether is for testing purposes only. Sorry, no Lambo.
For this exercise, that makes one unique address per voter on our election app.
We're going to need a directory for our bad-ass app to live in. So in GitBash let's type:
mkdir election
And hit Enter.
This will create a directory named election
Then type cd election
and hit Enter.
This will take us inside the directory named "election
" that we created.
Okay now we need to fire up our Truffle Framework, which has packs of pre-written code here so should save us a ton of work.
The pack or "box" we'll be using is the "pet-shop" box (Truffle has an Ethereum pet shop tutorial so that makes sense)
So in our Gitbash terminal let's type.
truffle unbox pet-shop
Hit Enter, and in a few moments you'll see:
Downloading...
Unpacking...
Setting up...
This might be fast or slow, depending on your computer. Mine is crap, so I watched
"Dances with Wolves" until it was done.
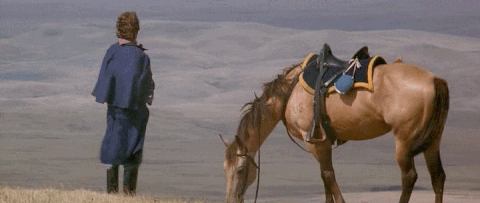
When it's done you should see the following:
Unbox successful. Sweet!
Now you can head on over to Sublime Text and click File -> Open Folder and navigate to the "election" directory you created.
The left hand section of the Sublime Text window should look like this:
Now back in GitBash we're going to create a new file in the contracts folder by entering the following command:
touch contracts/Election.sol
<-Note the capital "E" in the file name for better visibility (you'll thank me later).
The ".sol" is a file extension that indicates this file is a smart contract
If you're positive you did it correctly but still don't see the file as shown, restart sublime text and reopen the election folder and you should see it. If not then hit me up in the comments.
With the Election.sol file selected, we're now going to state, or as they say in the coding world, "declare" the version of Solidity we'll be using by typing this:
pragma solidity ^0.4.11;
and hitting Enter.
We'll then skip a line and declare our contract too by typing:
contract Election {
}
So now your screen should look like this:
Now we're going to define a function called "Election". This will tell Solidity that we're creating a "constructor". The constructor will hold a value, the name of our first candidate. We'll start by entering:
function Election () public {
}
The "public" part just basically means that we want it to run on the blockchain once we deploy the smart contract. Now our file should look like this:
Now inside (between the two curly braces) the function/constructor we declared , we're going to set a value to our candidate like this:
candidate = "Clinton";
heh.
Now let's see what we've got
Oh, a couple more things before we test and see that everything works so far.
We need to be able to both read and store our variable (candidate).
We do that with:
string public candidate;
entered just above our function line, so now we've got this:
We'll want to be able to interact with this thing on the blockchain, so we have to migrate it there. See the "migrations" folder just belows the "contracts" folder? If you twist that open you'll see a file inside called "1_initial_migration.js". We're going to copy and modify a bit of that code
Let's highlight and copy that. With the migrations folder highlighted, click File -> New File and paste the code in from the 1_initial_migration.js file
All we have to do is replace the word "Migration" in the three places with "Election", hit Save and name the file "2_deploy_contracts.js".
Now let's jump over to GitBash and type
truffle migrate
and hit Enter.
Again, it might take a moment or two depending on your machine. It should go through the migration process, you might or might not get some warnings but no worries it's fine as long as there are no actual errors. If you do encounter errors, let me know in the comments
Now lets try to open the Truffle console and interact a bit with the smart contract we made so far. In GitBash let's input:
truffle console
and hit Enter.
Shortly after that you'll see a prompt that looks like
truffle(development)>
Now let's see if this thing is working.
Let's enter:
Election.deployed().then(function(instance) { app = instance })
and hit Enter. If all goes well, you should see:
undefined
truffle(development)>
We'll get a bit more into detail in the next lesson, but for now just know that we just stored our app. Now we can try a command for example like:
app.address
and hitting Enter
You'll notice now that the series of numbers and letters corresponds with the first Ether address in Ganache! Woohoo!
You can test further by entering
app.candidate()
and Enter, and we should get the name of our candidate
'Clinton'
If you're having any trouble whatsoever with any of the steps so far please let me know.
Now that we've tested our app we can really start to flesh it out in the next step.
Hope you had fun and will back with Part 2 shortly!
Gifs by Giphy
Dances with Wolves (Film), Orion Pictures, 1990
This post has received a 0.24 % upvote from @drotto thanks to: @rudyardcatling.
You got a 2.59% upvote from @postpromoter courtesy of @doryumira!
Want to promote your posts too? Check out the Steem Bot Tracker website for more info. If you would like to support the development of @postpromoter and the bot tracker please vote for @yabapmatt for witness!
This post has received a 2.15 % upvote from @booster thanks to: @doryumira.
Congratulations! This post has been upvoted from the communal account, @minnowsupport, by doryumira from the Minnow Support Project. It's a witness project run by aggroed, ausbitbank, teamsteem, theprophet0, someguy123, neoxian, followbtcnews, and netuoso. The goal is to help Steemit grow by supporting Minnows. Please find us at the Peace, Abundance, and Liberty Network (PALnet) Discord Channel. It's a completely public and open space to all members of the Steemit community who voluntarily choose to be there.
If you would like to delegate to the Minnow Support Project you can do so by clicking on the following links: 50SP, 100SP, 250SP, 500SP, 1000SP, 5000SP.
Be sure to leave at least 50SP undelegated on your account.
Congratulations @doryumira! You have completed some achievement on Steemit and have been rewarded with new badge(s) :
Click on any badge to view your Board of Honor.
To support your work, I also upvoted your post!
For more information about SteemitBoard, click here
If you no longer want to receive notifications, reply to this comment with the word
STOP
Do not miss the last announcement from @steemitboard!