JavaScript - hasOwnProperty in `for-in` loops
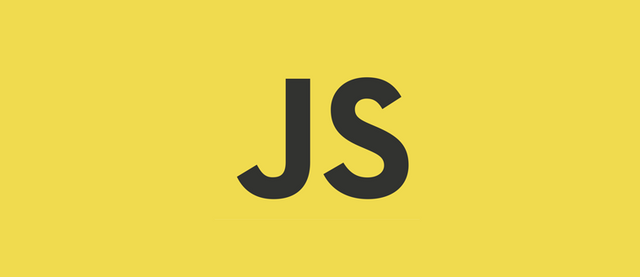
In most programming languages, iterating over an object (dictionary or map) is natively supported. and JavaScript supports it as well.
You've might seen iterating keys over object in JavaScript looks like this:
var obj = {
a: 1,
b: 2
};
for (var k in obj) {
if (obj.hasOwnProperty(k)) {
console.log(k); // prints a, b
}
}
Actually the following code might be working as well:
var obj = {
a: 1,
b: 2
};
for (var k in obj) {
// no hasOwnProperty check
console.log(k); // prints a, b
}
Then, You might say "Why we need additional useless hasOwnProperty
check?", and feel that this is useless and not natural. (Frankly, I thought so)
But this is not useless, this is very important to make your code safe and to make it always do right thing.
because JavaScript is too open to modify its inner implementations. Such as You can easily modify Object.prototype which is base prototype of all objects.
the following code is a simple example for messing up for...in
loop which has no hasOwnProperty
check by modifying Object.prototype:
var obj = {
a: 1,
b: 2
};
Object.prototype.haha = 3;
for (var k in obj) {
console.log(k); // prints a, b, haha
}
Someone (some libraries or some dependencies) could touch Object.prototype on your program context in anytime, Without hasOwnProperty
check, your for...in
loop might be iterating unexpected keys. So you need to make your for...in
loop safe using hasOwnProperty
check.
Object.keys()
In ES5+, You can use Object.keys()
to get its own enumerable keys of an object as well. and it will be much safer and easier to understand.
the following code is one with Object.keys()
:
var obj = {
a: 1,
b: 2
};
Object.prototype.haha = 3;
var objKeys = Object.keys(obj);
for (var k in objKeys) {
console.log(k); // prints a, b
}
It's simple and safe.
Hi @heejin,
Sorry, I'm confused a little:)
If I never change
Object.prototype
in my code, should i still check forhasOwnProperty
?I'll might going to use
Object.keys()
as it is really looks simple but first I want to understand if I'm in danger that something changes my Object without I'm asking for it, or not?Edit: I got it now, "You can easily modify Object.prototype" So it is me, who accidentally changing it! My question was lame:)
Yes and for example, If you write an Web Application, then you may use many libraries as dependencies.
and those libraries could change
Object.prototype
at anytime. So you'd better to use safer way.Good post. I used to run into bugs when I forgot to use the object's hasOwnProperty. Using Eslint in your preferred code editor is actually a good idea for catching this kind of bugs. I use atom for my work and have an atom eslint plugin that checks for errors.
Also checkout my new post on Promise Chaining
https://steemit.com/javascript/@codero/javascript-promise-chaining
Great js is very important in our programming life