SEC-S20W4 Decision Making in Programming: If-Then-Else
Hello everyone! I hope you will be good. Today I am here to participate in the contest of @sergeyk about the Decision Making in Programming. It is really an interesting and knowledgeable contest. There is a lot to explore. If you want to join then:
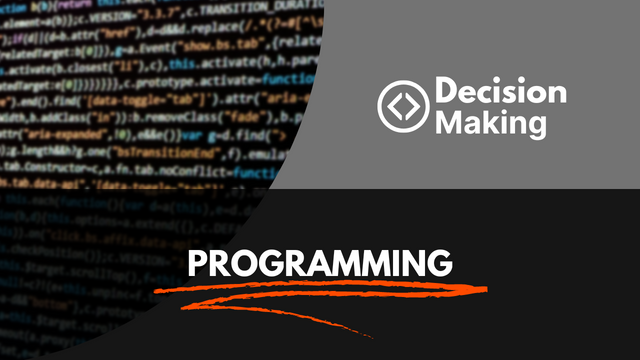
Find a universal and optimal solution to this problem.
I have found an optimal way to reach a specific number such as 100 or 13907 starting from 1 and by using only two operations adding 1 or multiplying by 2. In order to find the universal and optimal solution I will work backward from the target number to 1. It will help me to use the minimum steps to approach the target number in a universal way. Here is the reasoning process:
Backward Approach
I am going to use a reverse or backward method instead of figuring out how to grow from 1 to the target number. Let see how we can reduce the target number back to 1 using the reverse method. There are 2 rules in this regard:
- If the target is even the reverse of multiplication by 2 is division by 2 because we know that the previous number can be obtained by multiplying by 2.
- If the target number is odd the reverse of adding 1 is done by subtracting 1 from the number.
This method ensure that the most few operations will be used and we will be able to take advantage of faster growth with the help of the multiplication by 2 and adding 1 where needed.
Let me explain it with a short example of 100.
- Start with 100. It's even so divide by 2 → 100 ÷ 2 = 50.
- 50 is even so divide by 2 → 50 ÷ 2 = 25.
- 25 is odd so subtract 1 → 25 - 1 = 24.
- 24 is even so divide by 2 → 24 ÷ 2 = 12.
- 12 is even so divide by 2 → 12 ÷ 2 = 6.
- 6 is even so divide by 2 → 6 ÷ 2 = 3.
- 3 is odd so subtract 1 → 3 - 1 = 2.
- 2 is even so divide by 2 → 2 ÷ 2 = 1.
The operations in reverse are:
- Multiply 1 by 2 → 2.
- Add 1 → 3.
- Multiply by 2 → 6.
- Multiply by 2 → 12.
- Multiply by 2 → 24.
- Add 1 → 25.
- Multiply by 2 → 50.
- Multiply by 2 → 100.
This is a more optimal solution than just adding 1 repeatedly. It I have approached in just 9 steps to reach 100 from 1.
13709
Here is the reverse sequence of operations to reach 13709 from 1:
- Start with 13709. It is odd so subtract 1 → 13709 - 1 = 13708.
- 13708 is even so divide by 2 → 13708 ÷ 2 = 6854.
- 6854 is even so divide by 2 → 6854 ÷ 2 = 3427.
- 3427 is odd so subtract 1 → 3427 - 1 = 3426.
- 3426 is even so divide by 2 → 3426 ÷ 2 = 1713.
- 1713 is odd so subtract 1 → 1713 - 1 = 1712.
- 1712 is even so divide by 2 → 1712 ÷ 2 = 856.
- 856 is even so divide by 2 → 856 ÷ 2 = 428.
- 428 is even so divide by 2 → 428 ÷ 2 = 214.
- 214 is even so divide by 2 → 214 ÷ 2 = 107.
- 107 is odd so subtract 1 → 107 - 1 = 106.
- 106 is even so divide by 2 → 106 ÷ 2 = 53.
- 53 is odd so subtract 1 → 53 - 1 = 52.
- 52 is even so divide by 2 → 52 ÷ 2 = 26.
- 26 is even so divide by 2 → 26 ÷ 2 = 13.
- 13 is odd so subtract 1 → 13 - 1 = 12.
- 12 is even so divide by 2 → 12 ÷ 2 = 6.
- 6 is even so divide by 2 → 6 ÷ 2 = 3.
- 3 is odd so subtract 1 → 3 - 1 = 2.
- 2 is even so divide by 2 → 2 ÷ 2 = 1.
Reverse Operations to Reach 13709 from 1:
- Start with 1.
- Multiply by 2 → 2.
- Add 1 → 3.
- Multiply by 2 → 6.
- Multiply by 2 → 12.
- Add 1 → 13.
- Multiply by 2 → 26.
- Multiply by 2 → 52.
- Add 1 → 53.
- Multiply by 2 → 106.
- Add 1 → 107.
- Multiply by 2 → 214.
- Multiply by 2 → 428.
- Multiply by 2 → 856.
- Multiply by 2 → 1712.
- Add 1 → 1713.
- Multiply by 2 → 3426.
- Add 1 → 3427.
- Multiply by 2 → 6854.
- Multiply by 2 → 13708.
- Add 1 → 13709.
It was a long number and it was difficult to reach to it without using the reverse method in minimum steps. But now I have reached starting from 1 to 13907 in just 20 steps. So in this way we can easily reach to any number in minimum steps. This is the universal and optimal method to reach the target number.
What is the purpose of Boolean variables? Why are they called that?
Boolean variables are used to store true or false values. These values are often represented by 0 which is used for the false and 1 which is sued for the true. They help us to manage the decisions and flow of the program. We commonly used them in the conditional structures, loops and logical operations.
Key purpose of Boolean Variables
- Conditional Logic: Boolean variables are essential in the conditional statements such as
if
,else
andswitch
. They help to determine whether a particular block of code should be executed or not.
bool isRaining = true;
if (isRaining) {
cout << "Take an umbrella!" << endl;
}
Here the variable isRaining
is bool variable which is initially true and it checks the condition of the rain and execute the related code.
- Loop Coontrol: Booleans can be used to control the loops such as
while
offor
. The loop continues to run as long as the condition is false and it terminates when the condition becomes true according to the given example.
bool done = false;
while (!done) {
// Some operations...
done = true; // Loop exits when 'done' becomes true.
}
- Flags: Boolean variables are also used as flags to signal specific conditions in a program. For example we can set a flag to indicate whether a task has been completed or not.
bool taskCompleted = false;
// Perform task...
taskCompleted = true;
Here initially the variable taskCompleted
is false and when the task is completed we call it again with true value that will tell us the task has been completed.
- Simplifying Complex Logic: Boolean variables also help to simplify the complex problems by converting them into smaller parts. This improves the readability as well as maintainability of the code.
Why Are They Called Boolean?
It is really interesting to know why these are called Boolean because they could have any other name as well but why they are called as Boolean? What is the reason behind this specific name? So do not worry I am going to open this mystery here.
Long story short Boolean variables are associated with the name of the George Boole. He was an English mathematician and logician. He gave the foundation of the Boolean algebra in the mid of the 19th century. He is also known as father of Boolean logic. Boolean algebra deals with the binary values (0, 1) or (true, false). And most interesting thing is that these binary values are the basis of the modern digital computer logic. All the machines understand command and data in the binary numbers 0 and 1. Everything which we write in computers they convert it into binary numbers and then they understand it.
Boolean variables are a key tool in the programming languages. They play role in the decision making and in controlling the flow of the program.
What is the purpose of a conditional operator? What types exist?
As the name is conditional operator
so we can guess these are the operators which are used in some conditions. The purpose of a conditional operator is to provide a simple and short way to make the decisions based on the conditions. These are specifically beneficial in situations where we use an if - else
statement. Conditional operators allow to choose between two values or results depending upon the condition.
Types of Conditional Operators
Ternary Operator (
? :
)- It is the most commonly used type of the conditional operators. It is used to check a condition and returns one value from the two based on the condition whether it is true or false.
- It takes three operands such as a condition, a value if the condition is true and a value if the condition is false.
Syntax: condition ? value_if_true : value_if_false;
First of all the condition is written and then a question mark sign ?
is used and then the true condition is written and after that a colon :
is used and after that colon another condition is written.
C++ Example
int a = 10, b = 20;
int max = (a > b) ? a : b; // If 'a' is greater than 'b', max is assigned 'a', otherwise 'b'.
cout << "Maximum value: " << max << endl;
Here:
int max = (a > b)
is the condition- If it is true the value will be
a
- If the condition is false then the value will be
b
As we have taken a = 10
and b = 20
where the condition is false so the value b
will be returned as an answer.
Logical Operators (&&
. ||
, !
)
As the name itself tells these are the operators which are used to define the logic. Logical operators are used to create complex conditions by combining the simple ones. They are used with conditional statements specifically with an if
statement. We can also use them inside ternary operators to check more than one conditions at once. I have already explained these logical operators in my previous post and in this current post I will again explain them in a similar way.
AND Operator
This operator allows us to check two conditions at once and it returns true
when both the conditions are true otherwise false. The truth table of AND operator is given below:
A | B | A && B |
---|---|---|
0 | 0 | False |
0 | 1 | False |
1 | 0 | False |
1 | 1 | True |
From the above table we can see that if two values are true then it will return true otherwise false.
Example:
if(a > b) && (c<d) {
return true;}
{else return false;}
So in this way the logical operators check different conditions at once and return true or false value accordingly. (5 > 3) && (4 < 6)
returns true because both conditions are true.
OR Operator
This operator allows us to check two conditions at once and it returns true
when at least one condition is true otherwise it will return false. The truth table of OR operator is given below:
A | B | A OR B |
---|---|---|
0 | 0 | False |
0 | 1 | True |
1 | 0 | True |
1 | 1 | True |
From the above table we can see that if at least one value is true then it will return true otherwise false.
Example:
if(a > b) || (c<d) {
return true;}
{else return false;}
So in this way the logical operators check different conditions at once and return true or false value accordingly. (5 > 7) || (3 < 9)
returns true because the second condition is true.
NOT Operator
This operator allows us to reverse the value of a boolean expression. It turns true
into false
and vice versa. The truth table of NOT operator is given below:
A | !A |
---|---|
True | False |
False | True |
From the above table we can see that it converts the value from true to false and similarly from false to true.
Example:
!(5 > 3) returns false because 5 > 3 is true and NOT negates it and converting it to say (5<3) which is not true so this is the reason it is returning false value.
Relational Operators (>
, <
, >=
, <=
, ==
, !=
)
These operators compare two values and then return true or false. They are commonly used inside the conditional expressions as part of the if else
. They can also be used along with the ternary operators.
Examples:
Greater than (
>
): It checks if one value is greater than other value.
bool result = (a > b); // True if a is greater than b
Less than (
>
): It checks if one value is less than other value.
bool result = (a < b); // True if a is smaller than b
Less than or equal to (
<=
): It checks if one value is less than from the other or equal to the other.
bool result = (a <= b); // True if a is less than or equal to b
Greater than or equal to (
>=
): It checks if one value is greater than from the other or equal to the other.
bool result = (a >= b); // True if a is greater than or equal to b
Equality (
==
): This relational operator is used to check if two values are equal to each other.
bool result = (a == b); // True if a is equal to b
Not equal to (
!=
): This checks if one value is not equal to other value.
bool result = (a != b); // True if a is not equal to b
Conditional operators allow to write the code in a concise way for simple decision making. For example ternary operators eliminates the need for the multi line if else
statement. And you will love it to know that I call it the short form if if else
statement.
Example without Ternary Operator
int max;
if (a > b) {
max = a;
} else {
max = b;
}
Example with Ternary Operator
int max = (a > b) ? a : b;
It is how ternary operators reduces the number of lines of code. Without ternary operators the same logic was written in 6 lines but with the ternary operators the logic was written in just a single line.
Write a program to check if you’ve subscribed to 100 users.
Here's a C++ program that checks if you have subscribed to 100 users by reading the current number of subscriptions and comparing it to 100. I will get an input from the user to know the number of users he has subscribed to and then I will compare that value with 100. And based on the input of the user I will print the message to tell whether the user has subscribed to 100 or less or more than 100.
C++ Program
#include < iostream >
using namespace std;
int main() {
int subscriptions;
// Ask the user for the current number of subscriptions
cout << "Enter the number of users you've subscribed to: ";
cin >> subscriptions;
// Check if the user is subscribed to 100 users
if (subscriptions == 100) {
cout << "You have subscribed to exactly 100 users!" << endl;
} else if (subscriptions > 100) {
cout << "You have subscribed to more than 100 users." << endl;
} else {
cout << "You have subscribed to less than 100 users." << endl;
}
return 0;
}
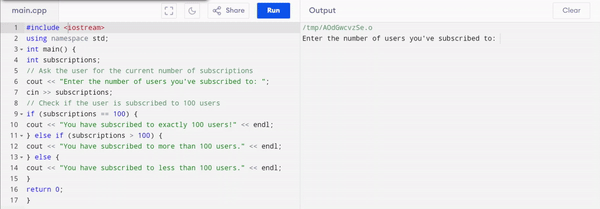
Explanation:
- The program asks the user to input the number of users they have subscribed to.
- It then checks:
- If the number of subscriptions is exactly 100.
- If the number is greater than 100.
- If the number is less than 100.
- Depending on the condition it gives the output.
Example Output:
If the user enters:
100
, the output will be:You have subscribed to exactly 100 users!
105
, the output will be:You have subscribed to more than 100 users.
95
, the output will be:You have subscribed to less than 100 users.
This program helps you easily check if you have reached exactly 100 subscriptions.
Write a program to check whether you have more posts, more subscribers or more Steem Power on steemit.com.
This is another interesting task which is related to our steemit profile. It is very simple to perform. The approach which I am going to use is to get input of the posts, subscribers and steem power from the user. and then I will compare all the values using conditional structures and will return the respective block of code. I will use if else if
statements to check different conditions. Moreover at the same time to compare more than one values as there are 3 values I will use logical operators according to their need.
C++ Program
#include < iostream >
using namespace std;
int main() {
int posts, subscribers;
double steemPower;
// Get inputs from the user
cout << "Enter the number of posts you have: ";
cin >> posts;
cout << "Enter the number of subscribers you have: ";
cin >> subscribers;
cout << "Enter your Steem Power: ";
cin >> steemPower;
// Compare the values
if (posts > subscribers && posts > steemPower) {
cout << "You have more posts than subscribers and Steem Power." << endl;
} else if (subscribers > posts && subscribers > steemPower) {
cout << "You have more subscribers than posts and Steem Power." << endl;
} else if (steemPower > posts && steemPower > subscribers) {
cout << "You have more Steem Power than posts and subscribers." << endl;
} else if (posts == subscribers && posts > steemPower) {
cout << "You have the same number of posts and subscribers, which is more than your Steem Power." << endl;
} else if (posts == steemPower && posts > subscribers) {
cout << "You have the same number of posts and Steem Power, which is more than your subscribers." << endl;
} else if (subscribers == steemPower && subscribers > posts) {
cout << "You have the same number of subscribers and Steem Power, which is more than your posts." << endl;
} else {
cout << "There is a tie among posts, subscribers, and Steem Power." << endl;
}
return 0;
}
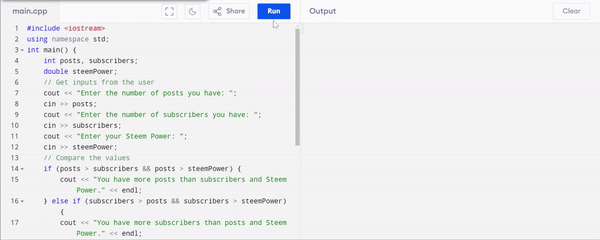
I have declared 3 variables:
posts
to store the number of posts.subscribers
to store the number of subscribers.steemPower
to store the amount of steem power
I have declared posts
and subscribers
as integers because all the these values are the whole numbers. But steemPower
maybe in decimal so I have declared as double
.
When the user is entering the values of the variables the program is applying the defined conditions on them and then returning an appropriate message accordingly.
In my case currently I have 4294 posts, 539 followers or subscribers and steem power is more than 4k so I have entered all these values as user input. The program told me that You have more posts than subscribers and Steem Power.
It compared all the values and returned the appropriate answer according to the user input. Similarly using this program anyone can check if their number of subscribers, posts or steem power is greater than others.
Given two numbers, write a program to determine whether their product or their sum is greater.
The structure and scenario of this program is same with the previous but the only difference is of the conditions and calculations. I will get 2 numbers from the user and I will not fix them in the program because if I fix the number in the coding then we will not be able to change it on run time. So to compare and see different results I will get the input from the user.
After that I will I will calculate the sum and product of those two numbers. Then I will use if
else
statements to check the sum and the product. If the sum of the numbers will be greater then my program will return the sum is greater otherwise if the product is greater then it will return the product of the numbers is greater than sum.
C++ Program
#include < iostream >
using namespace std;
int main() {
int num1, num2;
// Get inputs from the user
cout << "Enter the first number: ";
cin >> num1;
cout << "Enter the second number: ";
cin >> num2;
// Calculate the sum and the product of the two numbers
int sum = num1 + num2;
int product = num1 * num2;
// Compare the sum and product
if (product > sum) {
cout << "The product (" << product << ") is greater than the sum (" << sum << ")." << endl;
} else if (sum > product) {
cout << "The sum (" << sum << ") is greater than the product (" << product << ")." << endl;
} else {
cout << "The sum and product are equal (" << sum << ")." << endl;
}
return 0;
}
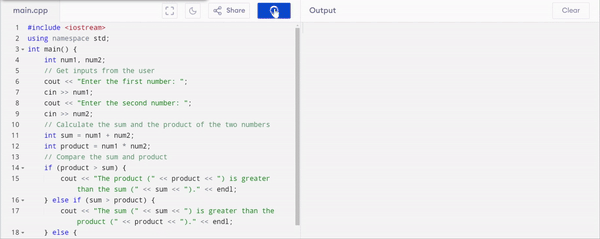
Here:
- I have initialized two integer numbers as
num1, num2
. - Then the program is asking the user to enter the first and second number
- After calculation of the sum and product the program is returning the related message to the screen.
At first I have entered 5 and 2 the program after calculations returns that the product (10) is greater than the sum (7). At the second attempt I entered 5 and 1 the program then returned me the sum (6) is greater than the product (5). In this way we can check any 2 numbers through this program and can determine whether their sum or product is greater than the other.
Given two house numbers, determine if they are on the same side of the street (assuming all even numbers are on one side, and all odd numbers are on the other).
As the professor has already indicated to us that the houses having even house numbers are at the one side but odd numbers are on the other side. So if the user inputs two house numbers which are even then the houses will be on the same side. And if the numbers are odd then the houses will also be on the same side. Another condition is that if the user inputs two numbers from them one is even and one is odd then the houses will be in opposite side.
In this program we have to use some conditional statements to check the entered number whether it is an even number or odd number. I will use modulus
operator %
to check the number is even or odd because if the modulus gives 0 remainder then the number is even otherwise the number will be odd.
After checking the nature of the numbers the program will proceed next to tell whether the houses are on same side or opposite side.
C++ Code
#include < iostream >
using namespace std;
int main() {
int house1, house2;
// Get inputs for two house numbers
cout << "Enter the first house number: ";
cin >> house1;
cout << "Enter the second house number: ";
cin >> house2;
// Check if both house numbers are on the same side of the street
if ((house1 % 2 == 0 && house2 % 2 == 0) || (house1 % 2 != 0 && house2 % 2 != 0)) {
cout << "Both houses are on the same side of the street." << endl;
} else {
cout << "The houses are on opposite sides of the street." << endl;
}
return 0;
}
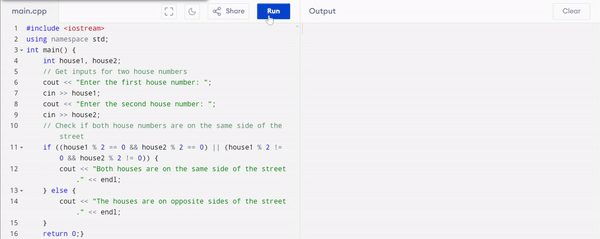
Here:
- I have initialized two variables
house1, house2
as integer because the house numbers are the whole numbers. These variables will be used to store the house numbers given by the user. - Then the program is accepting two integer house numbers from the user.
- After the user has input the house numbers the program checks if it is an even number or odd number with the help of the modulus operator.
- After the determination of the numbers the program is executing the relevant block of code and displaying the respective message on the screen.
Given a number, determine if it is true that it has more than four digits, is divisible by 7, and not divisible by 3.
After carefully reading and understanding the question I got to know that I have to write a program which checks the number for the 3 conditions such as:
- It has more than four digits.
- It is divisible by 7.
- It is not divisible by 3.
So there is the need to apply three conditions which will check the entered number. And then the program will return if the number meets the mentioned conditions or not. I will use cmath
library to count the digits by using log10
.
#include < iostream >
#include < cmath > // for log10 to count digits
using namespace std;
int main() {
int number;
// Get input from the user
cout << "Enter a number: ";
cin >> number;
// Condition 1: Check if the number has more than four digits
bool moreThanFourDigits = (abs(number) > 9999);
// Condition 2: Check if the number is divisible by 7
bool divisibleBy7 = (number % 7 == 0);
// Condition 3: Check if the number is NOT divisible by 3
bool notDivisibleBy3 = (number % 3 != 0);
// Check if all conditions are satisfied
if (moreThanFourDigits && divisibleBy7 && notDivisibleBy3) {
cout << "The number meets all the conditions." << endl;
} else {
cout << "The number does not meet all the conditions." << endl;
}
return 0;
}
Here:
- I have intialized a
number
as integer. - Then the program is checking different conditions according to the requirements.
- The first condition is checking if the entered number has more than 4 digits.
- The second condition is checking if the entered number is divisible by 7.
- Then the third condition is checking if the number is not divisible.
- Then there is the final condition which checks all the conditions at once and if all the conditions are true it is printing that
the number meets all the conditions
otherwise it is printingThe number does not meet all the conditions.
Which digit of a two-digit number is larger—the first or the second?
It is a simple program which will accept two digit number and then I will separate both the digits of the number with the help of the quotient and remainder. Then I will apply condition on each digit of the number. The condition will take both the numbers and it will check whether the first digit is greater than the second digit.
C++ Program
#include < iostream >
using namespace std;
int main() {
int number, firstDigit, secondDigit;
// Get input from the user
cout << "Enter a two-digit number: ";
cin >> number;
// Extract the first and second digits
firstDigit = number / 10; // First digit is the quotient when divided by 10
secondDigit = number % 10; // Second digit is the remainder when divided by 10
// Compare the two digits
if (firstDigit > secondDigit) {
cout << "The first digit (" << firstDigit << ") is larger than the second digit (" << secondDigit << ")." << endl;
} else if (secondDigit > firstDigit) {
cout << "The second digit (" << secondDigit << ") is larger than the first digit (" << firstDigit << ")." << endl;
} else {
cout << "Both digits are equal." << endl;
}
return 0;
}
Here:
- I have taken three integers
number
,firstDigit
andsecondDigit
. number
is storing the 2 digit number entered by the and then thefisrtDigit
variable will store the first digit of the number and similarly thesecondDigit
variable will store the second digit of the number.- Then the program is asking for a two digit number from the user.
- The user enters a two digit number.
- In order to get the first digit the program is diving the entered number by 10 and the first digit is the quotient.
- The second digit is the remainder when the digit is divided by the 10 with modulus operator.
- After that the program is checking whether the first number is great or second and similarly whether the first number is smaller or second.
- If a user enters both digits of the number same then it will say both numbers are equal.
Conditional structures are very important which help us in the decision making and we can use them to solve the complex problems. We use them in different situations as we have seen a number of tasks in the above section where we have used if else
statements.
Disclaimer: I have used Programiz compiler for the compilation of the code. All the screen recordings have also been taken from Programiz.
I invite @josepha, @bonaventure24 and @enamul17 to join this contest.
Upvoted! Thank you for supporting witness @jswit.
You have done so well and thanks for inviting me. I will try and take part also. I like how you have presented your first answer. Good luck to you.
try to participate, do not mind that there are so many tasks, they are simple. There is also a lot of text in the answers - you can answer shorter. And some of my puzzles are still unsolved.
0.00 SBD,
0.24 STEEM,
0.24 SP,
0.00 TRX
Okay sir, I will do well and participate. Thanks
Thank you so much dear fellow for the appreciation.
X Promotion: https://x.com/stylishtiger3/status/1842088663032660414
Thank you team 1 and especially @chant for the support.