SEC-S20W5 Iteration in Programming: For, While, and Do-While Loops
Hello everyone! I hope you will be good. Today I am here to participate in the contest of @sergeyk about the Iterations in Programming. It is really an interesting and knowledgeable contest. There is a lot to explore. If you want to join then:
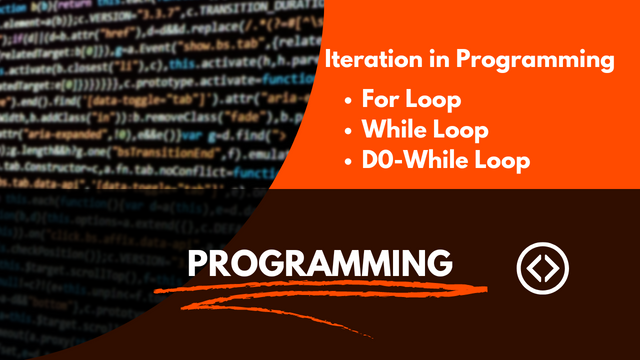.png)
Why are loops necessary in programming? Which type of loop did you like the most?
Loops are the most important elements is programming. They allow us to execute a block of code repeatedly based on the pre defined conditions. Loops help us to automate the tasks. We can avoid code redundancy. A simple code is more efficient then the program which includes redundant code. So instead of writing the same code again and again we can use loops.
Here are the important points which make the loops important in programming:
Automation of Repetitive Tasks: Loops save the time and efforts. Because the loops automate the repetitive tasks. For example: If we want to print the number from 1 to 100 or we want to calculate the sum of elements in a list then without writing the same code again and again we can simply use loops. We can a block of code once and we can execute it many times.
Handling Dynamic Inputs: There are many real world problems in which the data is not static. We need to process user input as well as data from the sensors. Sometimes we need to get information from the file or a database where the number of the items in the data are not known. And it is where loops allow us to manage the data dynamically and in an efficient way.
Efficient Resource Management: Loops allow us to manage the resources such as time and efforts in an efficient way. If we do not use loops then we have to write the same code again and again. And it will make the code bulky and unmanageable. Moreover loops make great use of the memory and processing power. It allows the computers to handle repetitive tasks. So the loops optimize the performance while reducing the complexity of the code.
Scalability: Loops make the code scalable. As the data grows in size loops allow to iterate this data systematically. For example: If we need to process thousands or millions of records in a database loops help us to iterate it. We can easily scale our program to handle the large data sets without changing the logic of the code.
Decision Making and Flexibility: Loops provide flexibility. All those scenarios in which number of iterations depend upon the dynamic conditions loops provide flexibility such as while processing user requests until an exit command. In order to manage unpredictable scenarios we use
while
anddo-while
loops.
- Parallel Processing: In our daily life problems we often encounter concurrent and parallel processes which we want to solve with programming. So loops help us in the parallel processing. They help to distribute and execute the code concurrently. Loops help to distribute the workload across all the processors.
Types of Loops
Again in this week I have alrerady explain this question in one of the homewroks of this week. But I will explain it again as well to support my arguments related to my preference of the loop. There are 3 types of loops which are given below:
- For Loop
- While Loop
- Do While Loop
For Loop
This loop repeats a block of code at a set number of times. It is used when we exactly know how many times we want to iterate our code. It has simple structure which includes intialization, condition and increment or decrement. And the syntax is written in a single line of code.
Syntax:
for (initialization; condition; increment/decrement) {
// Code to execute repeatedly
}
Initialization: This step is executed only once at the beginning. It is used to declare and set an initial value for a loop counter.
Condition: This condition defines that the loop will run as long as the condition is true. Once it becomes false the loop will stop.
Increment or Decrement: This part adjusts the loop counter after each iteration. If we use increment operator then it increments the value of the loop counter after each iteration and If we use decrement operator then it decrements the value of the loop counter after each iteration.
Example
![]() | ![]() |
---|---|
Here:
- I have intialized an integer variable i as
int i = 0
- I have given a condition for the number of iterations as
i < 5
- As I have started from 1 and I have to reach <5 so I need to increment the variable after each iteration so I have used increment operator as
i++
.
This loop prints the numbers from 0 to 4. We can customize the iterations by changing the condition. Further we can change it to work in the backward direction. We can also maintain the increment and decrement values.
While Loop
A while loop is used when we do not know the number of iterations. But we want to repeat the code as long as a condition is true. The condition is checked before each iteration of the loop.
Syntax:
while (condition) {
// Code to execute repeatedly
}
Condition: This conditions defines at much extent the program will be executed. As long as the pre defined condition is true the program continue to iterate. And when the condition is false the program is terminated. If the first condition is false then the program will never run.
Example
![]() | ![]() |
---|---|
Here:
The loop starts with
i = 0
and checksif i < 5
.After each iteration
i
is incremented by 1 untili
reaches 5. When the condition becomes false then the loop stops its iterations.
Do-While Loop
A do-while loop is similar to the previously discussed while loop. The only difference is of checking the condition. In this loop the condition is checked after the execution of the block of the code. And it ensures that the code will run at least once either the condition is true or false.
Syntax:
do {
// Code to execute
} while (condition);
Condition: It has the same condition like while loop that the code will continue to run until the condition is true. But the condition is checked after the execution of the code. The code will run at least once even the first condition is false.
Example
![]() | ![]() |
---|---|
Here:
The loop starts by printing
i = 0
. And on the next line it incrementsi
by 1.After printing it checks the condition whether
i < 5
. The loop continues untili
reaches 5.
My Preference
Personally I like for loop to use practically to perform the repetitive tasks. From the day one of loops practice I am preferring for loop. It is a versatile loop. It has a consize structure as you can see from the above examples and types of the loops. It is the best option to choose when the number of iterations are fixed and known.
When we are working with the arrays to handle the data we can use for loop to execute iterations according to the data of the array. If we want to get more than one inputs from the user then except writing the code again and again we can define for loop for the number of inputs. Similarly if we have an array which have specified data and we want to print the data of the array then we can again use for loop tor print the data using the index.
For loop allows us to control the iteration in an efficient way. So for loop is very useful when the data is finite. It is ideal to use in the precise iteration tasks such as processing the list, generating the sequences or iterating over a fixed period of time.
Explain both conditions in words. Which one is better and why?
Here is my explanation for both the conditions to determine if the buildings are on the same side of the street. Then I will compare both the conditions and after that I will tell which one is better than other.
Condition 1:
if(n % 2 == m % 2)
Explanation:
n % 2
calculates the remainder whenn
is divided by 2.- If
n
is evenn % 2
will be 0 and ifn
is odd thenn % 2
will be 1. - The same applies for
m
. - This condition checks whether both
n
andm
give the same remainder when divided by 2. - If both are even means remainder is 0 or both are odd means remainder is 1 then the condition will evaluate to true. It will indicate that both buildings are on the same side of the street.
Condition 2:
if(n % 2 == 0 && m % 2 == 0 || n % 2 == 1 && m % 2 == 1)
Explanation:
- This condition is a combination of two separate checks:
n % 2 == 0 && m % 2 == 0
checks if bothn
andm
are even.n % 2 == 1 && m % 2 == 1
checks if bothn
andm
are odd.
- The overall condition will be true if either both numbers are even or both are odd.
Comparison of the Two Conditions:
Complexity:
- The first condition (
n % 2 == m % 2
) is simple and concise. It achieves the same goal in one line. - The second condition is more complex. It involves additional comparisons which make it more complex.
- The first condition (
Readability:
- The first condition is easier to read and understand at a glance. But not for all only for someone who is familiar with the concept of checking evenness or oddness.
- The second condition can be harder to follow because it involves two separate checks. It involves logical operators as well.
Performance:
- Both conditions are efficient in terms of performance for this context. But the first condition may be faster due to fewer operations. It has one modulo operation and one comparison operation.
On the whole we can say that the first condition (if(n % 2 == m % 2)
) is better than the second condition. Because it is simple, more readable. And it implements the same logic as compared to the second condition in an efficient way. In the programming and coding clarity and the consizeness is very important and it counts a lot for choosing a method as well as a programmer. And first conditions leads in all these aspects.
Output the number 33 exactly 33 times using each type of loop.
It is really interesting task to define the same logic and getting the same output by using all types of loops.
For Loop
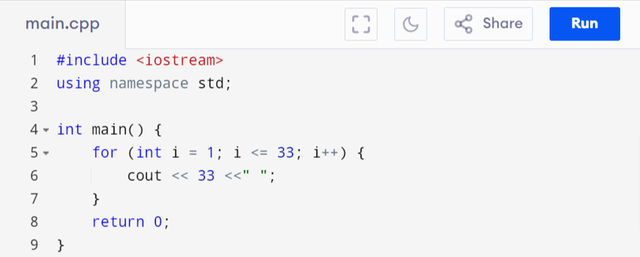
Here is the C++ code to print the number 33. I have defined the condition that the printing of 33 number starts from 1 and the loop continues to print the number 33 until it becomes 33.
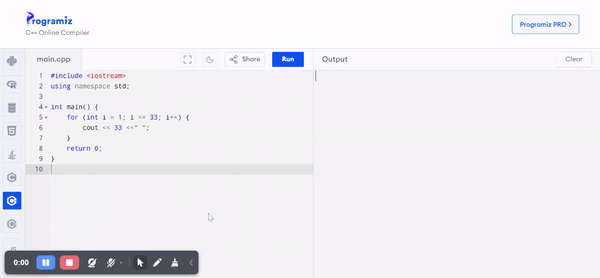
While Loop
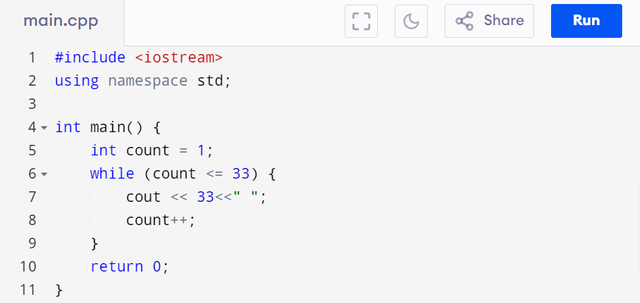
Here is the C++ code to print 33 by using while loop 33 times. The while
loop runs as long as the condition count <= 33
remains true or as long as the loop prints 33 times the number 33.
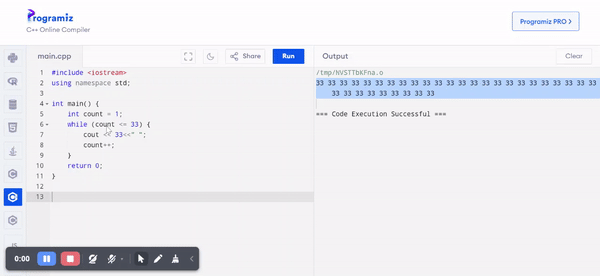
Do-While Loop
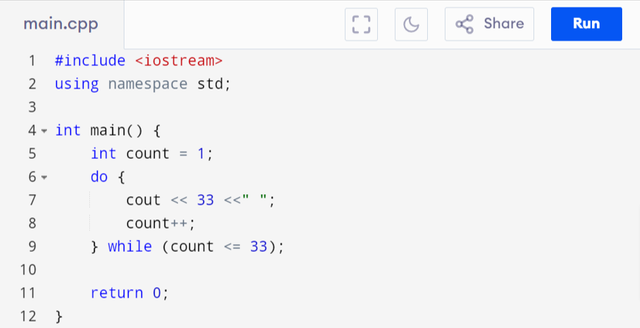
In the do-while
loop the code runs at least once whether the condition is true or false. The above do-while loop is printing 33 times the number 33.
Output numbers from 11 to 111
I will perform this task with the help of for loop
. As we need to start printing from 11
so I will start the loop from 11 such as int i = 11
and then I will terminate the loop until at 111. Each time the value is increasing by 10. such as 11+10= 21, 21+10, 31, 31+10 = 41. After the increment of 10 it becomes 51 and we need to skip this number ans so on we have to add 10 each times.
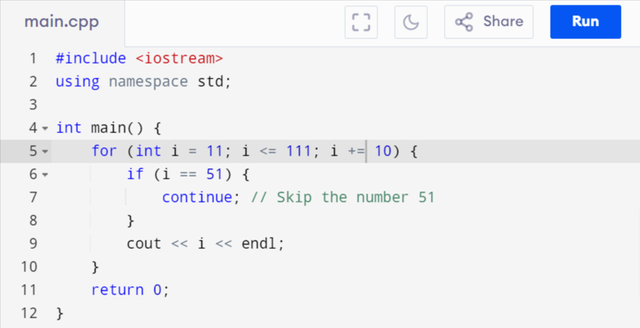
Here is the C++ code to print the required numbers. In this I have skipped 51 number from the list. And it will print all the numbers from 11 by incrementing 10 up to 111.
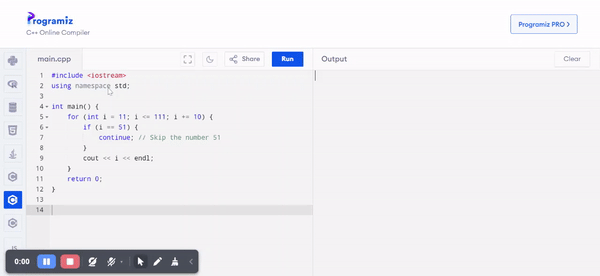
Here is the complete implementation of the above code which is printing all the required numbers.
Here:
- The
for
loop starts at 11. - There an increment of 10 each time until it reaches 111.
- The
if (i == 51)
the the condition skip this number from the block and moves to the next. - All other numbers except 51 are being printed from 11 to 111.
Output the multiplication table for a random number
We can make a table of any given number with the help of the loops. I will use for loop to output a table with the help of the program in C++.
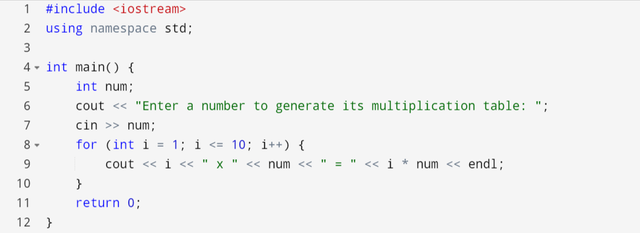
Here is the code to generate multiplication table of any number. I have defined to get the number from the user for which we want to print the multiplication table. The program displays a message to the user to input the number. That number is stored in the variable num
. And then this variable is used to multiply with the numbers from 1 to 10 consecutively.
I have used a for loop. It is starting from 1 as in the multiplication table the table starts from the number itself. And the loop will end after 10 iteration and in this way the table will also be printed.
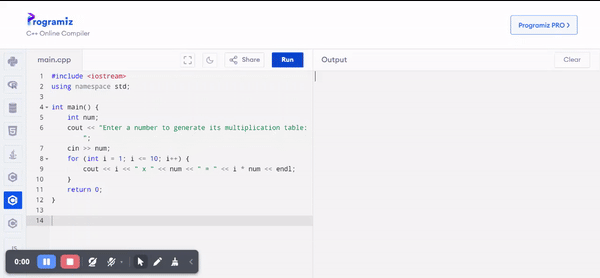
Output the squares of numbers: 1, 4, 9, 16, 25, 36, 49... 400.
According to my understanding of this question the professor is requiring the squares of the given numbers from 1, 4, 9, 16, 25, 36, 49... 400. The square of the number is the result of multiplying that number by itself.
- 1 is the square of 1
- 4 is the square of 2
- 9 is the square of 3
- 16 is the square of 4
- 25 is the square of 5
...
...
... - 361 is the square of 19
- 400 is the square of 20
So in order to calculate the squares of the numbers 1, 4, 9, 16, 25, 36, 49... 400 I have written the following C++ code:
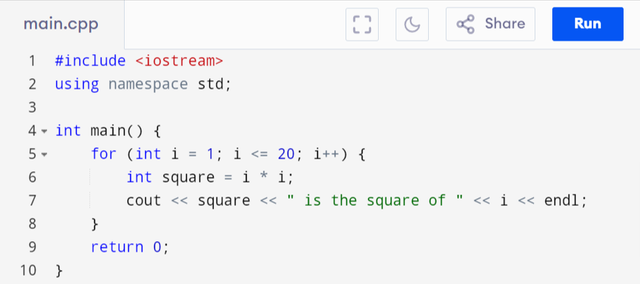
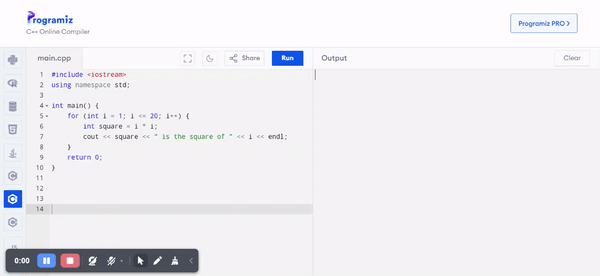
Output the powers of some number: 1, 2, 4, 8, 16, 32, 64, 128... less than 3000
We can output the powers of the given number using loops. According to the question requirement I need to output powers of the number starting from 1 until the output is less than 3000. Here is the C++ code for the question which will print the numbers until the value is less than 3000.
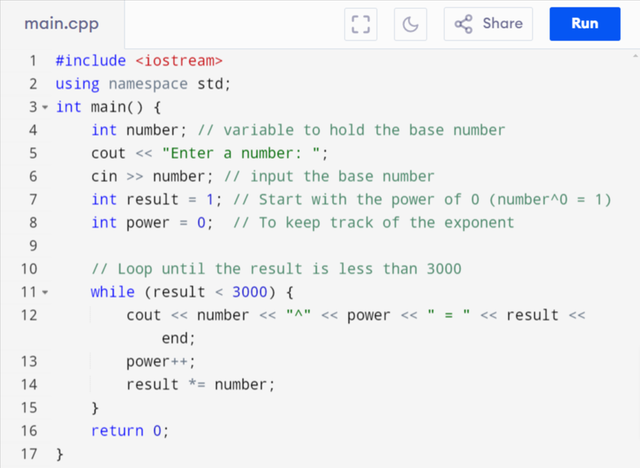
Here:
The program asks the user to input a number which is stored in the variable
number
. And this number will be used to hold the base number.Then I have initialized the variables.
result
variable starts at 1 such that n0 which is always equal to 1.power
starts at 0. It tracks the exponent.
Then there is the use of the while loop which continuously multiply the result by the number until the result is less than 3000.
Then there is the print statement and for each iteration it prints the current power and the result. And when the result reaches less than 3000 the program is terminated.
The output can be seen while using different numbers. It is just printing all the numbers starting from 1 to less than 3000.
How many even four-digit numbers are there that are not divisible by 3?
We can find it with the theoretical way by using some mathematical calculations as well as with the program. As we are dealing with programming so I will write a program that will find the total four digit even numbers. This program will also calculate even four digit numbers which are divisible by 3. Further the program will find the even four digit numbers which are not divisible by 3.
So here is the C++ code to perform this action and to find the solution of the question.
#include <iostream>
using namespace std;
int main() {
// Range of four-digit numbers
int first_even = 1000;
int last_even = 9998;
// Total even four-digit numbers
int total_even = (last_even - first_even) / 2 + 1;
// Smallest and largest even numbers divisible by 3
int first_div_by_3 = 1002;
int last_div_by_3 = 9996;
// Total even four-digit numbers divisible by 3
int even_div_by_3 = (last_div_by_3 - first_div_by_3) / 6 + 1;
// Even four-digit numbers not divisible by 3
int even_not_div_by_3 = total_even - even_div_by_3;
// Output the result
cout << "Total even four-digit numbers: " << total_even << endl;
cout << "Even four-digit numbers divisible by 3: " << even_div_by_3 << endl;
cout << "Even four-digit numbers not divisible by 3: " << even_not_div_by_3 << endl;
return 0;
}
I have used comments to explain all the code in a good way so that each variable and line of code can be understood easily by the reader.
Here:
I have defined the first and the last even number. The smallest four digit and the largest four digit even number is 9998.
I have used an arithmetic sequence formula to calculate the total four digit even numbers.
Then I have written the code to find the four digit even numbers which are divisible by 3. Again I have used the same arithmetic formula to find out the total number of the four digit numbers which are divisible by 3.
Then I have subtracted the even numbers divisible by 3 from the total number of even numbers to find out the even numbers which are not divisible by 3.
At the end the program will print the total four digit even numbers. It will filter four digit even numbers divisible by 3. And it will also filter the four digit even numbers which are not divisible by 3.
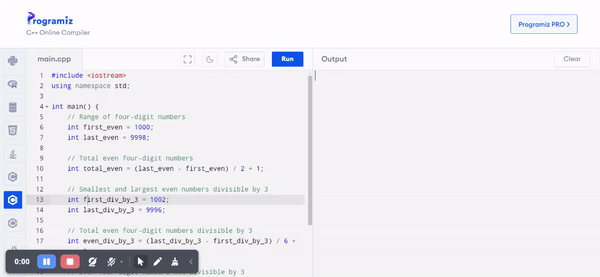
Disclaimer: I have used Programiz compiler for the execution and writing all the code. And all the screenshots of the code and gifs have been recorded from that website.
I invite @sahar78, @fombae and @kouba01 to join this contest.
Upvoted! Thank you for supporting witness @jswit.
X Promotion: https://x.com/stylishtiger3/status/1844800578771210709