SEC-S20W5 Iteration in Programming: For, While, and Do-While Loops
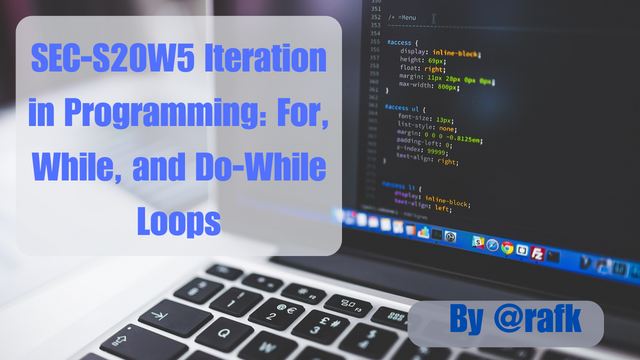
iteration in programming
Hi steemians , Happy to have this week's engagement challenge. I am delighted To be participating on this amazing contest. I hope you do as well. Let's begin.
Why are loops necessary in programming? Which type of loop did you like the most?
Cycles also referred to as Loops are a necessity in programming Which help to perform repetitive tasks more efficiently and effectively. They permit us to execute a block of code several times without having to write the same code repeatedly. This saves us time and , especially when working with large datasets or dealing with complex algorithms.
There exist different types of loops in programming Which are:
- For loops: Used in conditions where we know in advance how many times we are to repeat a block of code. They are often used to iterate over elements in a sequence, such as a list or array.
for (int i = 0; i < 5; ++i) {
// Code to be executed
}
- While loops: These are applicable when we don't know in advance how many times we need to repeat a block of code. They continue to execute as long as a certain condition is met until the condition is no longer true.
int count = 1;
while (count <= 5) {
// Code to be executed
++count;
}
- Do-while loops: These are similar to while loops, And works in a similar manner but they Make sure that the block of code will be executed at least once before the condition is checked.
do {
// Code to be executed
} while (condition);
Based on my personal reason, I Believe while loops to be the most valuable and powerful. They can be applicable in a wide range of situations, and they permit for more flexible control over the loop execution. Notwithstanding, it's important to use loops cautiously to avoid infinite loops, which can cause your program to crash.
⳾In task #8 from the last lesson, the condition for determining if buildings are on the same side of the street should have been written as:
if(n%2 == m%2)instead of:
if(n%2==0 && m%2==0 || n%2==1 && m%2==1)
Explain both conditions in words. Which one is better and why?
The two conditions are equivalent, but the first one is more concise and efficient.
Condition 1: if (n % 2 == m % 2)
This condition checks if the remainders of dividing n and m by 2 are equal. The other way around, it verify if both n and m are either even or odd. This is because the remainder of dividing any number by 2 is either 0 , if the number is even or 1 if the number is odd.
Condition 2: if (n % 2 == 0 && m % 2 == 0 || n % 2 == 1 && m % 2 == 1)
This condition strictly verify all possible combinations of even and odd numbers for n and m. It first checks if both n and m are even, then proceed to checks if both are odd.
Why the first condition is better:
The first condition is better because it is more concise and easier to read. It also avoids unnecessary calculations.
The second case verify all possible combinations of even and odd numbers, although only two of these combinations, both even or both odd are necessary for determining if the buildings are found the same side of the street.
Output the number 33 exactly 33 times using each type of loop.
For loop:
#include <iostream>
int main() {
for (int i = 0; i < 33; ++i) {
std::cout << 33 << std::endl;
}
return 0;
}
While loop:
#include <iostream>
int main() {
int count = 0;
while (count < 33) {
std::cout << 33 << std::endl;
++count;
}
return 0;
}
Do-while loop:
#include <iostream>
int main() {
int count = 0;
do {
std::cout << 33 << std::endl;
++count;
} while (count < 33);
return 0;
}
Explanation
For loop:
- Initialization:** The loop variable i is initialized to 0.
- Condition: The loop continues as long as i is less than 33.
- Body: The number 33 is printed to the console.
- Increment: The loop variable i is increased by 1.
- Repeat: Steps 2-4 are repeated until the condition is no longer true.
While loop:
- Initialization: The loop variable count is initialized to 0.
- Condition: The loop continues as long as count is less than 33.
- Body: The number 33 is printed to the console.
- Increment: The loop variable count is increased by 1.
- Repeat: Steps 2-4 are repeated until the condition is no longer true.
Do-while loop:
- Body: The number 33 is printed to the console.
- Increment: The loop variable count is increased by 1.
- Condition: The loop continues as long as count is less than 33.
- Repeat: Steps 2-3 are repeated until the condition is no longer true.
The main difference between the for
and while loops
is that the for loop's initialization, condition, and increment are all unified in a one header. The do-while loop, contrary, makes sure that the body of the loop is executed at least once before the condition is checked afterwards.
Output numbers from 11 to 111: 11, 21, 31... 101, 111, but skip the number 51 (using one type of loop)
For loop
#include <iostream>
int main() {
for (int i = 11; i <= 111; i += 10) {
if (i != 51) {
std::cout << i << " ";
}
}
std::cout << std::endl;
return 0;
}
Explanation
Initialization: The loop variable i is initialized to 11. This marks the starting point for the loop.
Condition: The loop continues as long as i is less than or equal to 111. This ensures that all numbers from 11 to 111 are included.
Check for 51: The if statement checks if the current value of i is equal to 51. If it is, the loop skips the rest of the body and proceeds to the next iteration.
Output: If i is not equal to 51, the value of i is printed to the console followed by a space.
Increment: The loop variable i is incremented by 10. This moves to the next number in the sequence of numbers that end in 1.
Repeat: Steps 2-4 are repeated until the condition is no longer true.
In essence, the loop iterates through the numbers from 11 to 111, skipping 51 and outputting the remaining numbers to the console.
Output the multiplication table for a random number, for example, for 0
In this task, I will be using the for loop,
defining where my multiplication table will end that is 10. I pick any random number let's say 6. And start the multiplication from 1 to 10.
#include <iostream>
#include <cstdlib>
#include <ctime>
int main() {
// Seed the random number generator
srand(time(nullptr));
// Pick a random number between 1 and 10
int randomNum = rand() % 10 + 1;
// Print the multiplication table for the random number
std::cout << "Multiplication table for " << randomNum << ":" << std::endl;
for (int i = 1; i <= 10; ++i) {
std::cout << randomNum << " x " << i << " = " << randomNum * i << std::endl;
}
return 0;
}
Explanation
iostream: The header is included for to define basic input/output operations.
cstdlib:
This header provides various utility functions, including rand and srand for random number generation.ctime:
This header is used for functions related to time, it is used to seed out the random number generator.Seed the random number generator:
srand(time(nullptr)):
This line seeds the random number generator using the current time. This ensures that the generated random numbers are different each time the program is running.Generate a random number:
int randomNum = rand() % 10 + 1:
This line generates a random integer between 1 and 10. The rand() function generates a random integer, and the % 10 operator takes the remainder of dividing that number by 10, resulting in a number between 0 and 9. Adding 1 to this result ensures that the random number is between 1 and 10.Print the multiplication table:
std::cout << "Multiplication table for " << randomNum << ":" << std::endl;:
This line prints a header indicating the multiplication table for the generated random number.for (int i = 1; i <= 10; ++i) { ... }:
This for loop iterates through the numbers from 1 to 10.std::cout << randomNum << " x " << i << " = " << randomNum * i << std::endl;
This line calculates the product of the random number and the current iteration value i and prints the result in a formatted string.
Output the squares of numbers: 1, 4, 9, 16, 25, 36, 49... 400.
#include <iostream>
int main() {
for (int i = 1; i <= 20; ++i) {
int square = i * i;
std::cout << square << " ";
}
std::cout << std::endl;
return 0;
}
Explanation.
Initialization: The loop variable i is initialized to 1. This marks the start of the loop.
Condition: The loop continues as long as i is less than or equal to 20. This ensures that the squares of numbers from 1 to 400 are calculated.
Calculate square: The expression i * i calculates the square of the current value of i. The result is stored in the variable square.
Output: The value of square is printed to the console followed by a space.
Increment: The loop variable i is incremented by 1. This moves to the next number in the sequence.
Repeat: Steps 2-4 will repeat until the condition is no longer true or it's false.
In essence, the loop iterates through the numbers from 1 to 20, calculates the square of each number, and outputs the result to the console. The for loop is a suitable choice for this task because we know the exact number of iterations needed (20).
Output the powers of some number: 1, 2, 4, 8, 16, 32, 64, 128... less than 3000.
#include <iostream>
int main() {
int power = 1;
while (power < 3000) {
std::cout << power << " ";
power *= 2;
}
std::cout << std::endl;
return 0;
}
Explanation
Initialization: The variable power is initialized to 1. This represents the starting point for the powers of 2.
Condition: The loop continues as long as power is less than 3000. This ensures that only powers of 2 less than 3000 are output.
Output: The current value of power is printed to the console followed by a space.
Update power: The value of power is multiplied by 2. It computes the next power of 2.
Repeat: Steps 2-3 will repeat until the condition is no longer true.
In essence, the loop begins with the power 1, then repeatedly multiplies it by 2 to get the next power of 2. The loop continues until the power are is above 3000. The while loop is a more applicable choice for this kind of task because we don't know for sure the exact number of iterations we need to perform to reach 3000. The loop continues until the condition is false.
How many even four-digit numbers are there that are not divisible by 3?
To find the number of even four-digit numbers that are not divisible by 3, we can apply the following approach:
Count all even four-digit numbers: There are 9000 four-digit numbers in total (from 1000 to 9999). Half of these are even, so there are 9000 / 2 = 4500 even four-digit numbers.
Count even four-digit numbers divisible by 3: For a number to be divisible by 3, the total of the digits of a number must also be divisible by 3. For even four-digit numbers, the last digit should be 0, 2, 4, 6, or 8. If the last digit is 0 or 6, the sum of the remaining three digits must be divisible by 3. Contrary if the last digit is 2, 4, or 8, the sum of the remaining three digits must be congruent to 1 modulo 3 meaning the gcd(a,m) is 1. Using combination, we can calculate that there are 1500 even four-digit numbers divisible by 3.
Subtract divisible numbers from total: To get the number of even four-digit numbers that are do not divide 3, we subtract the number of even four-digit numbers divisible by 3 from the sum number of even
four-digit numbers. We have 4500 - 1500 = 3000.
In essence, there are 3000 even four-digit numbers that are not divisible by 3.
Concluding, I will say it was an amazing task carrying out All the various iterations for the different cycles in programming.
Without hesitation, I would like to invite the following persons participate with me in this contest. @simonnwigwe, @chant, @fombae, @daprado1999
Cc: @sergeyk
Credit to: @rafk
replace the picture of your post, this is the same image from my post
Alright..