SLC21 Week5 - Programming Games&Puzzles
Assalamualaikum my fellows I hope you will be fine by the grace of Allah. Today I am going to participate in the steemit learning challenge season 21 week 5 by @sergeyk under the umbrella of steemit team. It is about Programming Games&Puzzles. Let us start exploring this week's teaching course.
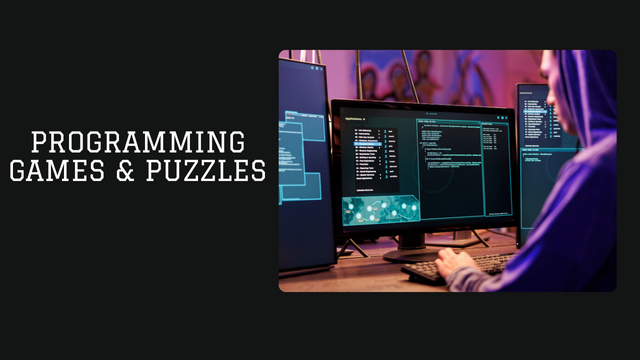.png)
Make the field longer, put ( в init()) on the edges, for example, of a pillar - # at a random distance of 0-2 from the edges of the map (field). The player must move between these pillars.
In the example given by the teacher the size
is 20. And now I will enhance this size from this limit to the greater limit. And then by following the requirement of the task I will add the pillars on the sides of the track. The pillars will be represented by the #
. And in between these pillars the player will be able to move and the player will not be able to move beyond these pillars.
For the implementation of the pillars on the corners I will modify the init()
function. And it will place the pillars (#
) at random distances (0-2) from the edges of the field. These pillars will act as boundaries that the player cannot pass through. Additionally we will update the move()
function to ensure the player cannot move through the pillars.
Explanation
Field with Pillars:
- Two pillars (
#
) are randomly placed near the edges of the field usingrand() % 3
to ensure their positions are within 0-2 cells from the edges.
- Two pillars (
Player Placement:
- The player (
@
) starts at the center but avoids starting on a pillar.
- The player (
Movement Restrictions:
- The
move()
function ensures the player cannot move into a cell containing a pillar.
- The
Increased Field Size:
- The field size is increased to 30 for better gameplay dynamics.
Now the size of the field has been increased and the pillars has been installed on the corners of the field. The player cannot move beyond beyond the pillars.
Complete the first task, make the field even longer, randomly place puddles on the field _. The player has to pass through these puddles without obstacles, only to change his appearance to %a wet player.
Currently the game field size
is 30 but according to the requirement we will change this size to a greater size. Now I will increase the field size by 10 and it will become 40 and it will be enough to accumulate the puddles on the field. If we place 10 puddles then there will be the remaining size of 30 for the player to move on the field where puddles are not present.
So to fulfil this task of adding puddles to the field and changing the player's appearance when stepping on them I have made the following changes and additions to the code:
Increased Field Length
- The field size has been increased to
const int size = 40;
to create a longer field for the gameplay.
Puddles on the Field
- Symbol for puddles: The
_
character is used to represent puddles on the field. - Random placement: Puddles are randomly placed so that they do not come on the same place in excess. The puddles are placed ina way that they do not overlap the pillars in the game.
Wet Player State
Player appearance changes to
%
: When the player steps on a puddle their appearance changes from@
which is the normal state to%
which shows the wet state.Condition to become wet:
When the player step in the puddles then thewet
flag becomestrue
. It means that the player has entered the puddle.Player display logic: The
display()
function checks thewet
state to render the player as%
:
Game Flow Adjustments
- The
move()
function now checks if the player's new position contains a puddle (PUDDLE
) and updates thewet
state accordingly. - Stepping on a puddle does not end the game but it visually changes the player's state.
Game Over Message
- A conditional check was added to recognize if the player quits the game while in the "wet" state.
Moreover the code was looking very bulky because of the comments so I have removed some comments and I have made the comments short and appropriate. And now the appearance of the player is changing when it is stepping into the puddle.
jump Add a jump (spacebar) - the player jumps one cell in the direction of his movement!!!
To add the jump feature whikle pressing the spacebar (' '
) allows the player to jump one cell in the direction of their last movement we need to make the following modifications:
Track Last Movement
- We will introduce a variable to track the player's last movement direction ('L' for left and 'R' for right). This will allow the player to jump in the correct direction.
Implement Jump Logic
In the move()
function, handle the spacebar (' '
) input to move the player in the last movement direction if valid.
But while this we need to be careful that jumping does not bypass collisions with pillars or ignore puddles.
1. Added char last_move
:
It tracks the player's last movement direction to determine jump direction.
2. Handled Spacebar Input:
It checks if the spacebar (' '
) is pressed and moves the player two cells in the direction of last_move
.
3. Boundary and Collision Checks:
It prevents jumping beyond the field limits or into a pillar.
4. Game Flow Adjustments:
We have updated the move()
function to include the jump functionality.
Now the player can jump over puddles or avoid obstacles by pressing the spacebar. It makes the game more dynamic.
the puddle disappears If the player jumps (through the space) into a single puddle - it dries up, when the player simply runs through the puddle - it does not dry up.
To make puddles disappear when the player jumps into them we need to modify the game logic. Specifically we will do these changes:
Track Jump Into Puddles:
- If the player jumps into a cell containing a puddle (
'_'
), the puddle will dry up (replaced by'.'
).
- If the player jumps into a cell containing a puddle (
Ensure Normal Movement Doesn't Affect Puddles:
- When the player walks (not jumps) through a puddle it remains as a puddle.
Update the
move()
Function:- Handle puddle drying only during a jump.
Dry Puddle on Jump:
- If the player lands on a puddle during a jump (
field[x] == PUDDLE
), the puddle is replaced with'.'
.
- If the player lands on a puddle during a jump (
Preserve Puddle During Walk:
- When the player moves normally through a puddle, it remains as a puddle.
Jump Direction:
- Maintains logic for left and right jumps based on
last_move
.
- Maintains logic for left and right jumps based on
This implementation ensures puddles only disappear if the player jumps into them and when it does not jump the puddle remaisn same as it can be seen in the video.
stones Complete the first task, make the field even longer, randomly place stones on the field a. The player cannot cross these stones, but must cross these stones.
To add stones ('a'
) to the field and ensure that the player cannot cross them while walking but can cross over them if jumping we need to modify the following parts of the code:
Randomly Place Stones (
'a'
):- We will randomly place stones on the field to ensure that they do not overwrite pillars or puddles.
Prevent Player from Moving through Stones:
- If the player tries to move onto a stone they will be blocked and cannot proceed.
Allow Jumping Over Stones:
- Jumping will allow the player to bypass stones just like they can bypass puddles during a jump.
Key Changes:
- Add Stones Randomly to the field.
- Prevent Movement if the player tries to walk into a stone (
'a'
). - Allow Jumping Over Stones by skipping over them during a jump.
Stone Placement:
- Stones are randomly placed on the field in a similar manner to puddles but using the
'a'
character. - Stones cannot overwrite existing pillars or puddles.
- Stones are randomly placed on the field in a similar manner to puddles but using the
Stone Collision:
- The player cannot walk through stones (
'a'
) and if the player attempts to move into a stone a collision occurs and the game ends.
- The player cannot walk through stones (
Jumping Over Stones:
- When the player jumps they can jump over stones just like they can jump over puddles meaning stones won't block the player during a jump.
- The player will see stones as
'a'
on the field. - If the player tries to move onto a stone the game will stop with a "Game Over" message.
- The player can still jump over stones using spacebar if there is enough room to jump over them.
statistics Add statistics: - number of moves, jumps, puddles, stones.
To track statistics such as the number of moves, jumps, puddles passed, and stones encountered we need to add variables that will count these actions throughout the game. These counters will be updated every time the player moves, jumps, or interacts with puddles or stones.
Key Changes:
- Move Counter: Tracks the number of moves made by the player.
- Jump Counter: Tracks the number of jumps made by the player.
- Puddle Counter: Tracks the number of puddles the player passes through.
- Stone Counter: Tracks the number of stones the player encounters.
We will update these counters in the move()
function based on player actions. After the game ends then we will display these statistics.
Key Updates for Statistics:
Counters Added:
move_count
: Counts the total number of movements.jump_count
: Counts the number of jumps.puddle_count
: Tracks the number of puddles the player passes through (not dried up by walking).stone_count
: Tracks how many times the player encounters stones.
Updating Counters:
move_count
is incremented whenever the player moves (either left or right).jump_count
is incremented when the player jumps.puddle_count
is incremented each time the player passes through a puddle.stone_count
is incremented each time the player encounters a stone.
Game End Statistics Display:
- At the end of the game, the statistics are printed showing the total number of moves, jumps, puddles passed, and stones encountered.
This ensures that the player receives feedback on how they performed throughout the game, keeping track of important actions such as jumping over puddles or encountering stones.
push A player can push one stone, no more.
To implement the functionality where the player can push a stone, we need to add a new interaction in the movement logic. The player should be able to push a stone by moving into it. But only if the next cell in the direction of movement is empty such as the player can push the stone to an adjacent space. The player can only push one stone at a time, and pushing a stone will count as a move.
Key Changes:
- Stone Pushing: If the player moves into a stone they will "push" the stone to the next empty space.
- Limits on Stone Pushing: The player can only push one stone at a time and only if the space ahead of the stone is empty.
We will need to adjust the move()
function to handle the logic of pushing the stone. Additionally we will ensure the player can only push one stone and cannot push the stone into an obstacle which can be a pillar or out of bounds.
Key Changes for Stone Pushing:
Stone Push Logic:
- The player can push a stone if the space next to the stone is empty (
.
). - The player can only push one stone at a time, marked by the
stone_pushed
flag. - If the stone is pushed, the stone is moved, and the player's current move is processed, skipping the usual move logic.
- The player can push a stone if the space next to the stone is empty (
Limits on Pushing:
- The player can only push a stone if the next space is empty (
.
). If the next space is blocked by an obstacle, the player can't push the stone further.
- The player can only push a stone if the next space is empty (
Game Statistics:
- The
stone_pushed
flag ensures that the player can only push a single stone once. You can expand this to allow the player to push another stone later by resetting this flag if needed.
- The
Now the player can push stones if possible but they are limited to pushing one stone at a time and it only works if there is space available to move the stone.
I invite @wirngo, @wuddi, and @chant to learn strings.
I have compiled all the code of the tasks in a famous online compiler which is OnlineGDB
Congratulations, your post has been upvoted by @scilwa, which is a curating account for @R2cornell's Discord Community. We can also be found on our hive community & peakd as well as on my Discord Server
Felicitaciones, su publication ha sido votado por @scilwa. También puedo ser encontrado en nuestra comunidad de colmena y Peakd así como en mi servidor de discordia
Thank you for participating, at first glance everything looks correct - I will give a detailed assessment of your work later.