SLC21 Week6 - Programming Games&Puzzles (part 2)
Hello Everyone
I'm AhsanSharif From Pakistan
Greetings to you all, hope you all are well and enjoying a happy moment of life with steem. I'm also good Alhamdulillah. |
---|
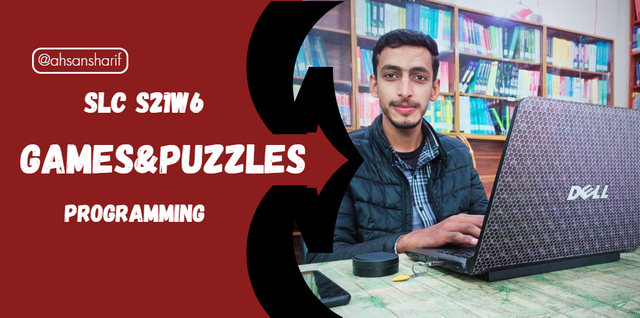
Design On Canva
There are many areas in the computer world where random numbers play an important role, some of which are as follows:
Simulations:
Random numbers are used in simulations, where they play an important role as real-world processes are modeled, such as weather forecasting and physics experiments.
Gaming:
Random numbers also play an important role in video games, where they determine elements such as enemy behavior, and loot methods, or even improve replayability.
Algorithm:
It is also used in algorithms, where it is used to take random samples or to find solutions to complex problems.
Procedural Content Generation:
Random numbers are also used in applications to create the texture or surface of the Earth or in 3D modeling.
Turning Pseudo-Random into More Random Ones
As we know computers work on deterministic algorithms and hence they cannot generate true randomness but they use mathematical formulas and generate pseudo-code numbers. If know the initial state (seed) then predict. There are some strategies to make these numbers more random, which are as follows:
Seeding With External Data:
In C or C++, if we use a constant seed, it generates a single same sequence, which is srand(), and if we use the function rand()
, that generates new random numbers every time. We can seed the generator with a dynamic value. If we want to display our current time, we use a srand(time(0))
. Because it changes over time, there will be a new random number for it at each point.
e.,g.
srand(time(0)); // Seed with the current time
int randomNum = rand(); // Generate a random number
TRNGs:
TRNG stands for True Random Number Generators. They are used for important applications such as cryptography. They are used to demonstrate physical phenomena that are truly random because they cannot be predicted by algorithms.
Entropy Sources:
There are many unexpected events in the world that we cannot predict, which can lead to randomness. As we see for example, user input including their mouse movements or keyboard timing is also used to seed random number generators for entropy, make more unpredictable.
I will generate the random number using C++ and the rand()
function and control it with srand()
.
Random Number Generator using rand()
:
The function is used because it ensures that the sequence of random numbers is different every time we run the program. This function srand(time(0))
seeds the random number generator with the current time.
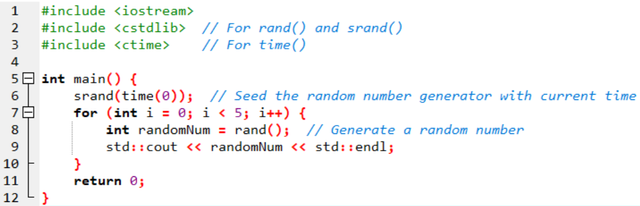
The rand()
function generates random numbers between zero and a max value. If we want it to generate numbers between one and one hundred, for example, we can use the up modulo operator to limit our range.
Example With range:
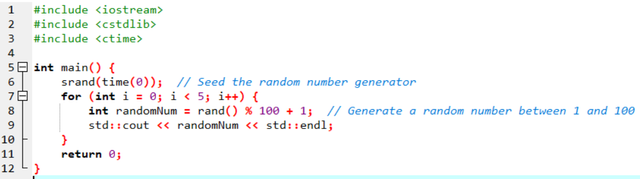
If we use a fixed seed, which is srand(10)
, then the random numbers will always be the same no matter how many times you run the program.
If we use srand(time)(0))
, we get a new sequence every time we generate it because it depends on the current time.
Here are the ways:
Simple Loop from 1 to N:
For filling the array with numbers from 1 to N here is the simple straightforward way.
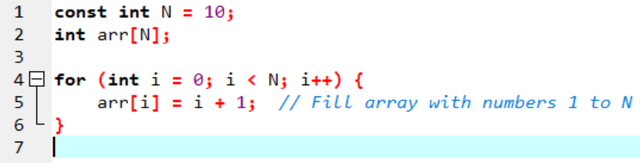
Filling in Reverse Order:
This method is the reverse of our first method. It fills the array with numbers from N down to one.
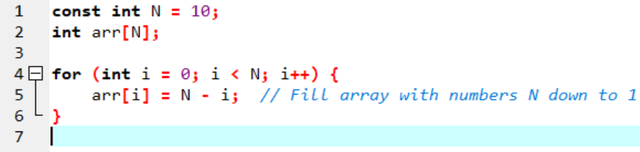
Filling with Random Numbers:
This is a method in which it does not guarantee unique numbers. It fills the array with random numbers from one to the N.
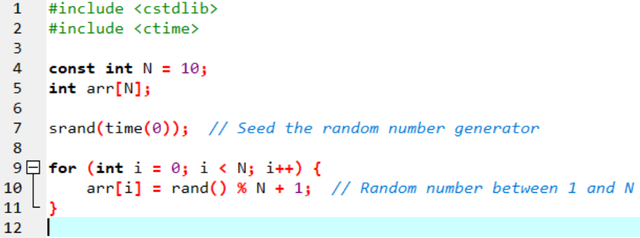
It fills its array with random numbers using the rand() function, but since there is no check for uniqueness, it can write the same number twice.
Brute Force Random Numbers:
With this method, we can check that our array is filled with different random numbers but it checks for duplicates.
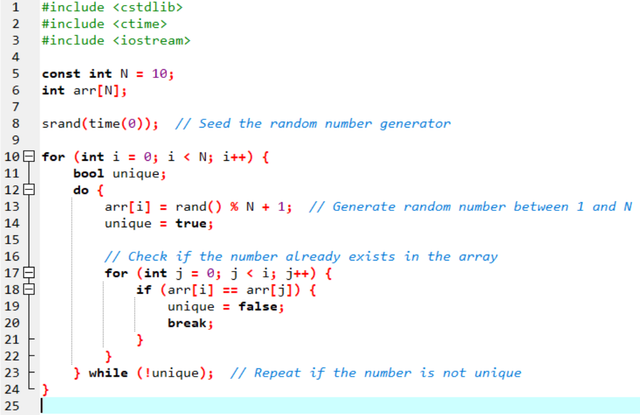
This is also the same method as the previous method that generates a random number, but here its function is that it checks the number to see if it is a duplicate. If the number is a duplicate, it generates a new number in its place and keeps checking until a unique value is found.
Shuffling a Pre-filled Array:
It fills the array from one to N in a sequence, then it shuffles it using an algorithm called the Fisher-Yates algorithm.
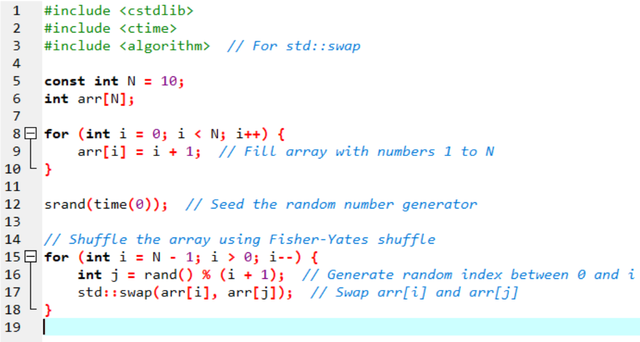
It efficiently shuffles all the numbers, guarantees that each number is unique, and makes the shuffle random.
In the methods we have studied earlier, to estimate the number of cards N, we need to know all of its operations, and how many operations it performs in each method. We can see here how operations increase as the number of cards N increases. Here we will briefly review it and mention the number of operations.
Simple Loop: This method of N iteration only runs a single loop for N cards. (One Operation per card)
Filling in Reverse: This method is similar to the first method, it runs the N iteration loop only once. (One Operation per card)
Filling with Random: It runs N times and each time it runs it generates a random number. It does not show the uniqueness. It is a basic N operation. (Random generation per card)
Brute Force Random: In this, a random number is generated for each card, and then we check if that number is already present in the array. This includes a loop inside it that grows with the size of the array. (Operation Roughly N2/2)
Shuffling a Pre-filled: In this it fills the array with a sequence and then swaps it N-1. Each swap generates a random number and involves swapping two elements. (operations: generating random numbers and performing swaps)
Estimated Operation
Ncards | Simple | Reverse | Random | Brute Force | Shuffling |
---|---|---|---|---|---|
5 | 5 | 5 | 5 | 12.5 | 10 |
6 | 6 | 6 | 6 | 18 | 12 |
7 | 7 | 7 | 7 | 24.5 | 14 |
8 | 8 | 8 | 8 | 32 | 16 |
9 | 9 | 9 | 9 | 40.5 | 18 |
10 | 10 | 10 | 10 | 50 | 20 |
This is a table that shows us how the size of our array grows with each operation. Simple methods grow linearly while Fisher Yates is efficient. Operations are growing linearly. And brute force grows quadratically which is inefficient.
This is what I understand to perform this task. Thank you so much for staying here. I would like to invite @abdullahw2, @josepha, @rumaisha to join this challenge.
Cc:
@sergeyk
Congratulations! This post has been voted through steemcurator09. We support quality posts, good comments anywhere and any tags.
It is best not to curator vote on Learning Challenge posts, as these posts are well supported by 01 02.
@waterjoe
The rules of steemitblog say that writings from the teaching team cannot be voted on
Terima kasih banyak teman atas undangannya, sesungguhnya aku sangat ingin berpartisipasi, tapi bagian kursus ini aku kurang paham.
Postingan anda sangat menarik dan penjelasan anda juga lengkap. Semoga sukses. ❤️