Learn Coding #3 - PHP - if - else - elseif
You often have cases where you want to execute php code if certain conditions are given. And other code if it's not. To implement this you use if , else and elseif.
How to use
Like stated in the last tutorial there are different types of variables. Today we need the boolean (The value can either be true or false)
$a = 5;
$b = 6;
$c = $a < $b; // $c = true
$c = $a > $b // $c = false
To clarify how an if statement is built:
$username = "smooms";
$enteredPassword = "password";
$databasePassword = getUserPassword($username);
// getUserPassword() would be a function call which will return the password of given username
// not realy realistic but it's enough for example purpouses
// how functions work will be shown in later episodes
if ($enteredPassword == $databasePassword) {
// code to execute if password is correct
} else {
// code to execute if password is wrong
}
So this is a small example how an if-else could look like. You will see that I used 2x =. In an if statement with 2 = the 2 values will be checked. If they are equal the statement returns true.
You can also use 3x =, then the datatype will also be checked. In our example, $enteredPassword has to be from the same type as $databasePassword (if 3 = where used)
Another ways to use if-else
If there is not much code involved in an if statement you can also do one liners.
Example:
$a = 5;
$b = 6;
$c = ($a < $b) ? $a : $b; // if true then $c = $a else $c = $b
Syntax: (condition) ? [what do if true] : [what do if false];
I often use this example inside HTML Forms. For autofilling fields. If you have a Login form it would be nice that if you enter your credentials wrong that your username keeps disappearing each time you submit the form. (I will show you in another tutorial how this works)
Another good example how to use if-else inside of HTML Code is a user contribution from robk on the php.net platform:
easy way to execute conditional html / javascript / css or
other language code with php if else:
<?php if (condition): ?>
html code to run if condition is true
<?php else: ?>
html code to run if condition is false
<?php endif ?>
A nice example because you often have to combine php with html.
And what about elseif?
Yes, there also are elseifs. They somehow work like elses. But it combines with if. An ifelse will be called if the previous if doesn't hold true. It would look something like that:
$a = 5;
$b = 6;
$c = 7;
if ($a > $b) {
echo $a + $b;
} elseif ($b < $c) {
echo $a + $b + $c:
} else {
echo 0;
}
// The output will be???? (Put your answer as a reply)
So you see. Theoreticaly you can chain as many elseif as you want.
A little bit of logic
In if and elseif it is possible to chain the conditions. For example:
$a = 5;
$b = 5;
$c = 7;
$d = 1337;
if ($a == $b && $c < $d) {
echo "This should show now." //because this is true
}
So now we have && which simbolizes a logical AND. Means that both querys have to be true in order to be completely true.
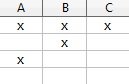
So A and B both have to be true in case for C to be true as well.
And also an example for OR:
$a = 5;
$b = 5;
$c = 7;
$d = 1337;
if ($a > $b || $c < $d) {
echo "This should show now." // And it also does. $a isn't bigger than $b, but $c is smaller than $d
}
So the two | symbolize the logical OR. Either one or both statements have to be true in order to be the if statement to be completely true.
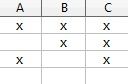
So if either A or B is true (or even both are true) then C is true too.
Some more things nice to know
!= corresponds to is not equal.
You can also use a ! to invert the result of a condition.
For example:
$a = 5;
$b= 6;
$c = !($a == $b); // $a == $b should return false, but through the ! the result is true. It would also work vise versa
Homework
Of course you have all read this post carefully and followed every step. So you have to reply only one thing I was asking for in this post. ;)
I was looking forward to write something about arrays and loops as well but that would make this post even longer than it is already. I will try to throw something out in the next days or next tuesday at the latest.
If you are new with my tutorials and don't know how to execute PHP code, then I encourage you to read this.
And of course feel free to follow @smooms for more and I appreciate every upvote and resteem to help other people learn to code PHP.
Since then and have a nice week.
@OriginalWorks
@cleverbot
@banjo
@steemprice BTC
@steem-untalented
$17449.27 USD/BTC
Volume: 52830.04 BTC ($921845790.32 USD)
Last Updated Tue Dec 12 23:56:49 2017
The @OriginalWorks bot has determined this post by @smooms to be original material and upvoted(1.5%) it!
To call @OriginalWorks, simply reply to any post with @originalworks or !originalworks in your message!
What do my bones have to do with my beliefs?
Thank you. That's a very nice thing to say.
Hello @smooms
We have given you our still tinnie-winnie upvote!
You have been determined by a human @smooms to be possessing of special gem. Do join the #untalented family!
Abolishing the conditioning attached to IQ tests e.g smart, dull, bum, average and you suddenly give every human a chance to shine!
So e.g if Bill Gates did Microsoft and i can do Macro-hard, arent we both genius? Oh, we both are! Showcase your talents regardless of its nature freely with us in #untalented. Relegate all reservations as flaws are allowed and we sift even the so-called nonsense to find sense therein.
#untalented is an ongoing historical curriculum with initiatives/contests, where each participant wins something. No losers! It is also a core branch of #steemgigs, so please join the SteemGigs community on discord already containing around 700 gifted steemian family members. See this URL for more info
https://steemit.com/steemit/@surpassinggoogle/steemit-s-untalented-is-in-beta-participate-freely-because-every-participant-in-this-contest-will-win-something-no-losers
and also this URL for the discord community
https://discord.gg/CGuPyyT
If you want to vote a witness, you can vote steemgigs too. Simply go to
https://steemit.com/~witnesses
and type steemgigs into the first search box. Stay awesome!
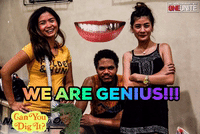
Just incase you find any level or form of gifts, talents, attempts at out-of-the-boxness, or any steemian low in confidence about their abilities, worth, etc; please don't let it slip emptily by.
Kindly call on me! Simply reply to any such post and add @steem-untalented or #untalented to your reply and i will be there to upvote, acknowledge, strengthen and encourage them.
This post has received gratitude of 0.93 % from @jout thanks to: @smooms.
This post has received a 0.63 % upvote from @drotto thanks to: @smooms.
Coding is one of my favorite things to do. What languages are you knowledgeable in?
Well I am a software engineer. There are some languages i am able to code in. But Job related it's mainly php, java, python and c#.
I'd love some python tutorials and avanced java tutorials too if you ever consider it.
What I wanted to do was some Python Steem Library stuff. But my internet is realy slow at the moment and for that I have to update Python. But if you follow my blog you will see when I start something with Python. :)
I don't know of any good python editors. I have eclipse for my javascript stuff. Any suggestions?
I had one a few years ago. But you had to pay for that. So eclipse is my way to go too there. Doesn't know the name of the other one though. But luckily there are Plugins for every purpouse for eclipse. But I also need to update Python in my system. And actualy my Internet speed is at about 32 kb/s and that would take days since I would habe downloaded Python.
@smooms payed 3.57 SBD to @minnowbooster to buy a stealth upvote.
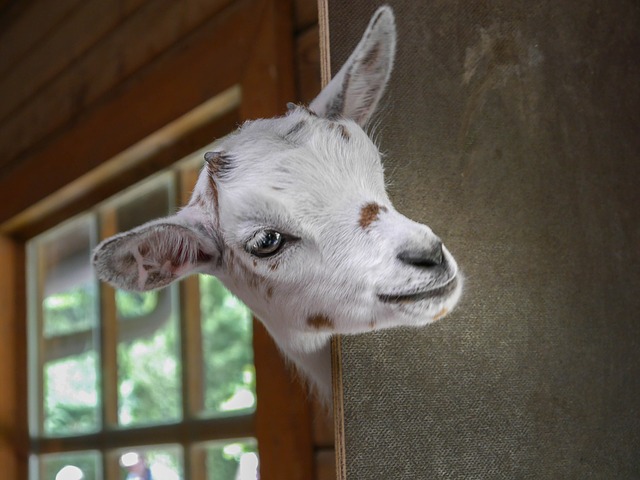
transaction-id 9f2fe900ae18cab4f945ba2fca91c8e860caab3b
@stealthgoat
Nice one :)
https://steemit.com/coding/@gaottantacinque/rasmus-lerdorf-php-inventor-in-milan